- Expand the Unity's project file
Mecanim.zip
which was created in
"Unity5: Use a Humanoid Model created by yourself (move)".
Rename the folder to "mecanim2/".
-
Based on the "PlayerMove.unity" scene in the above project,
create a "PlayerMoveAnim.unity" scene.
- File -> Open Scene -> Open the "Assets/Scenes/PlayerMove.unity" Scene
- File -> Save Scene as ... -> Save Scene as "Assets/Scenes/PlayerMoveAnim.unity".
-
Prepare motion animation to use with Humanoid Character.
I will use Standard Assets of Unity here.
Assets -> Import Package -> Characters -> With the "All" selected -> Import
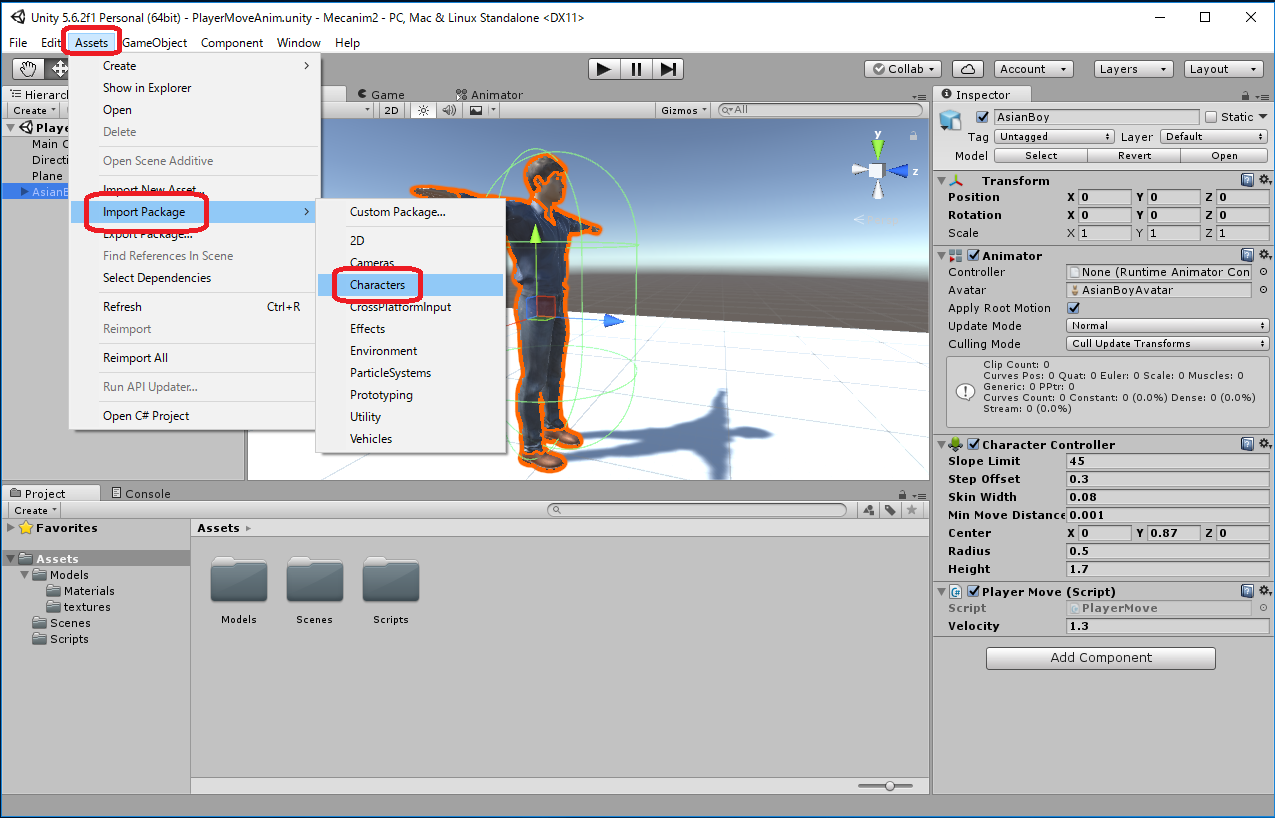
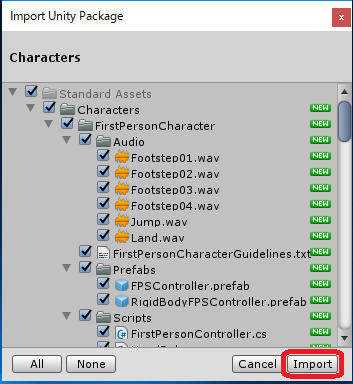
Behavior animaions for the Humanoid Character are imported to the
"Assets/Standard Assets/Characters/ThirdPersonCharacter/Animation/"
in the project window.
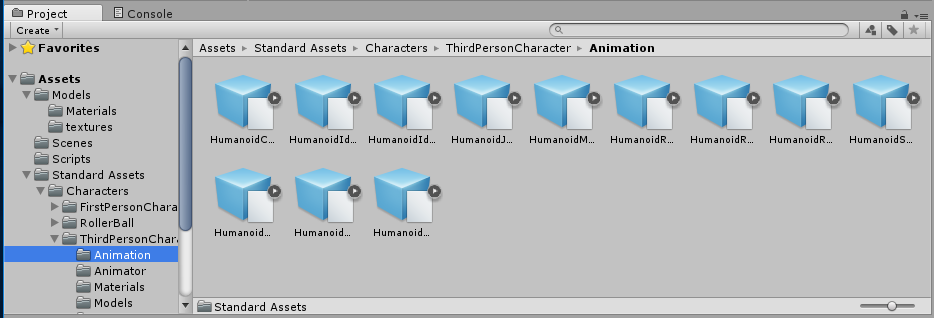
- Add Animator Controller component to the AsianBoy in the Hierarchy.
- Create the "Assets/Animators/" folder in the Project window.
Right click in the Assets -> Create -> Foler -> rename to "Animators"

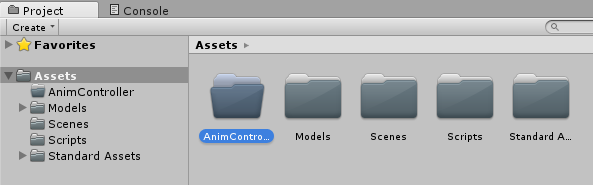
- Create Animator Controller in the "Assets/Animators" folder in the Project window,
and rename i to "AsianBoyAnimController".
Right click in the Assets -> Create -> rename to "Animator Controller"
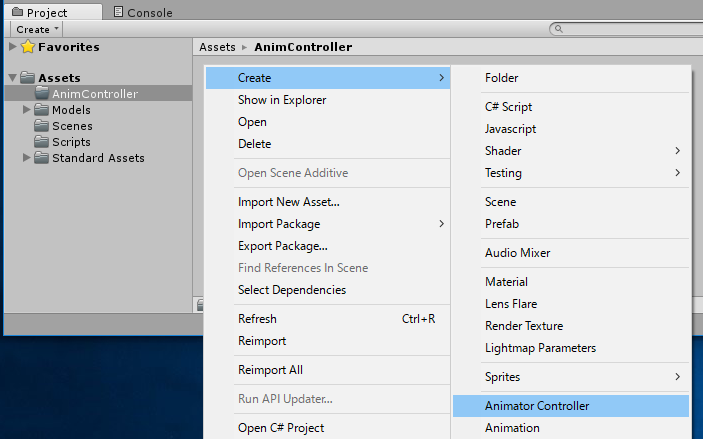
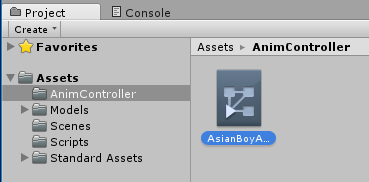
- Show the Animator window.
the above menu bar -> Windoow -> Animator
Animator window will be shown.
"Layers" and "Parameter" can be switched between display and non-display.
But if it is hided, let's switch to display.
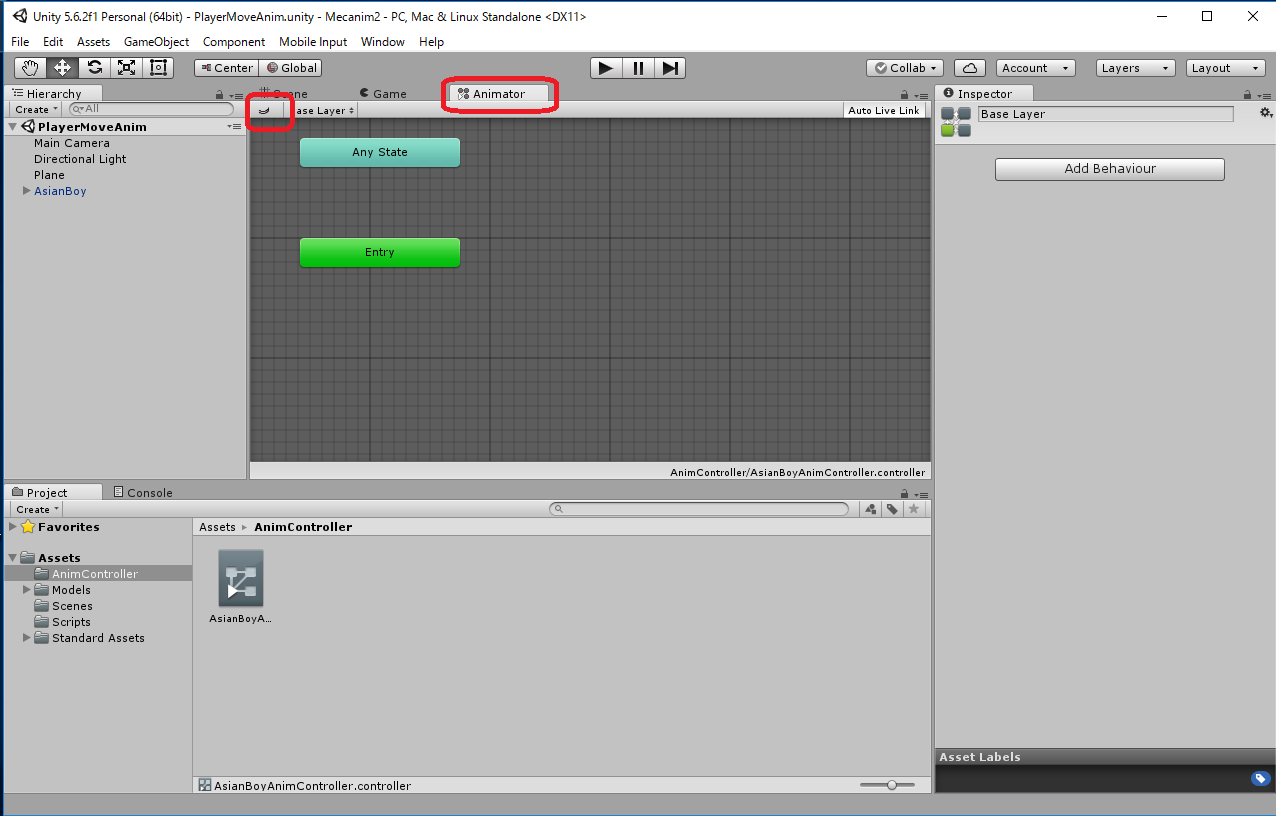
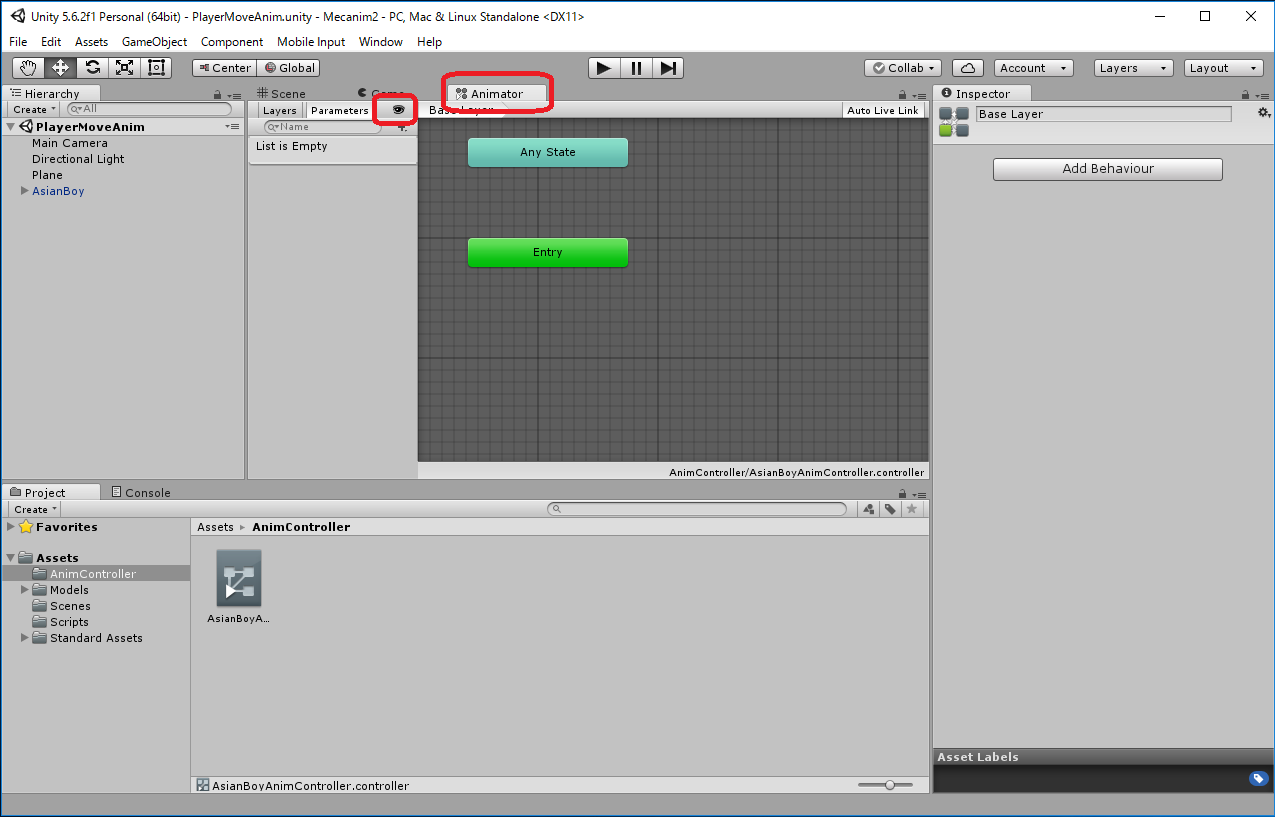
- What is displayed in the Animator window are "Character State" and "State Transition".
- Add the "Idle" state in the Animator windows.
Set Motion in this state to "HumanoidIdle".
This Motion (= Animation) is included in the "Standard Assets/Characters/ThirdPersonCharacter/Animation/".
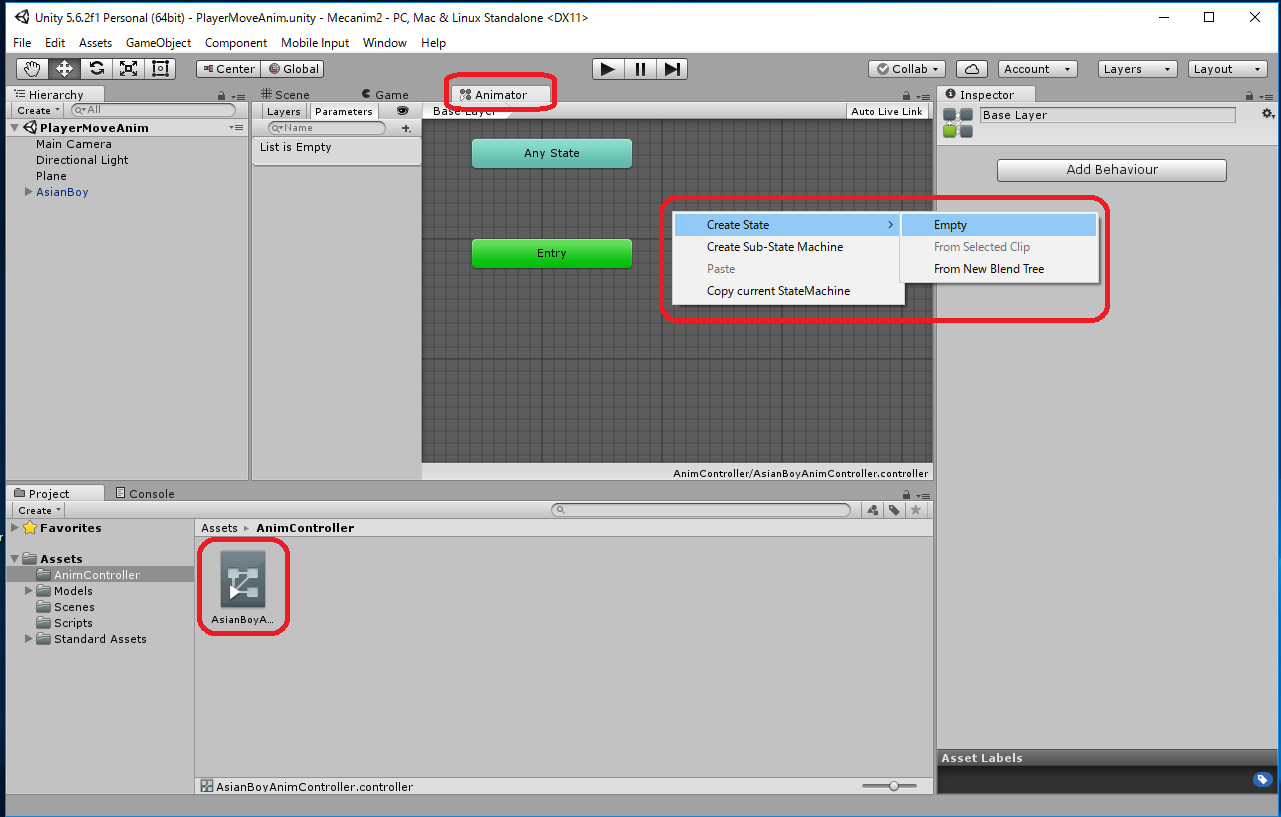
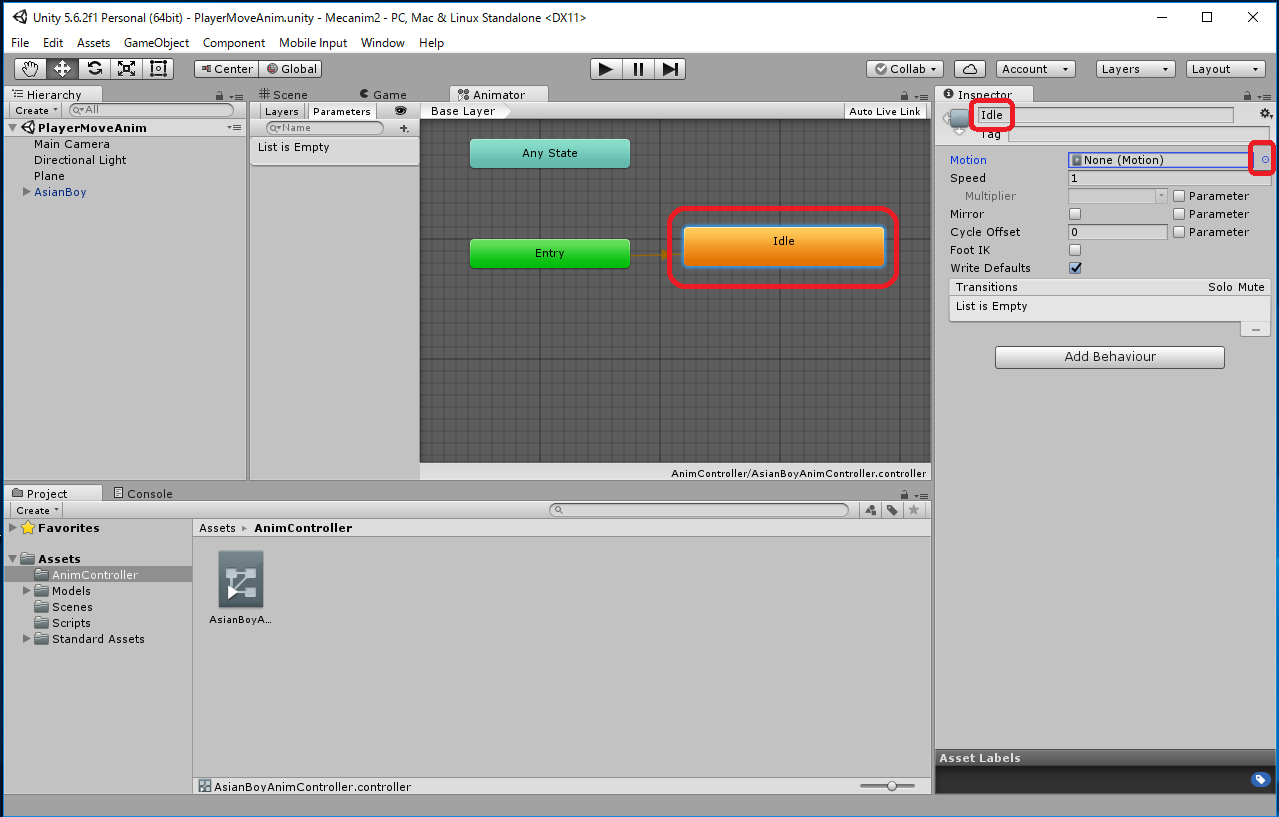
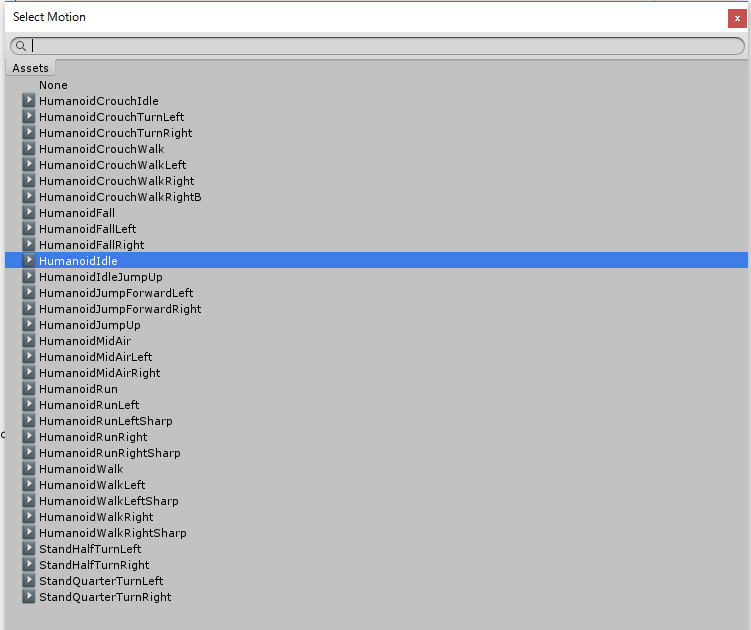
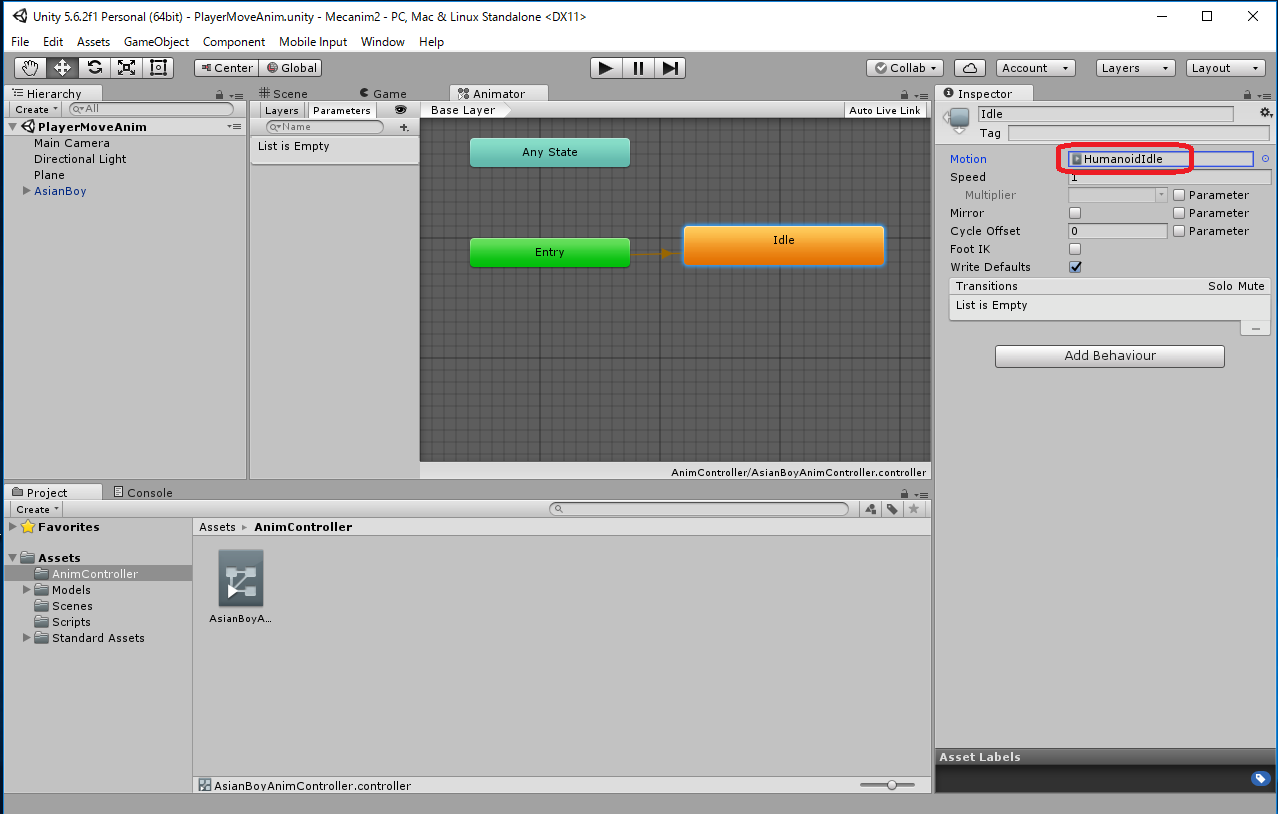
- Add "Walk" state in the Animator window.
Set Motion in this state to "HumanoidWalk".
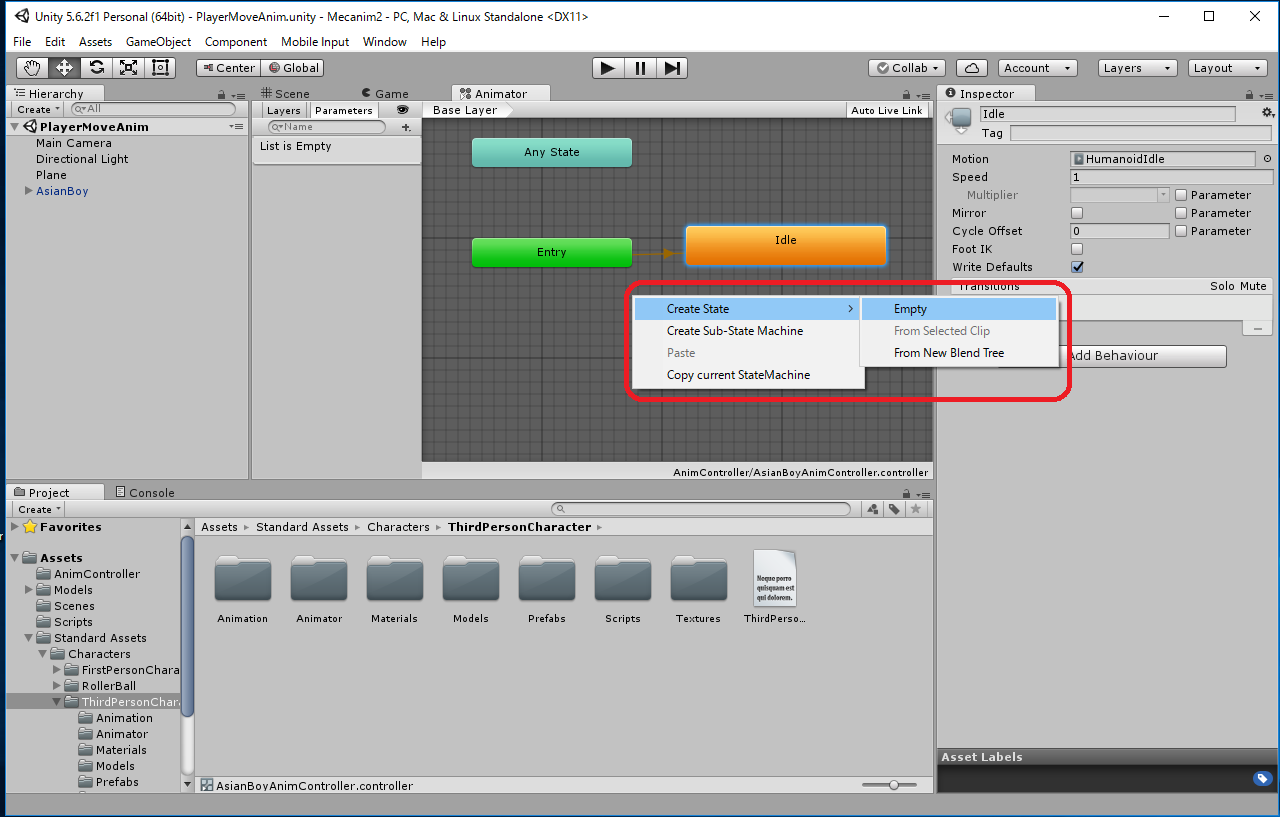
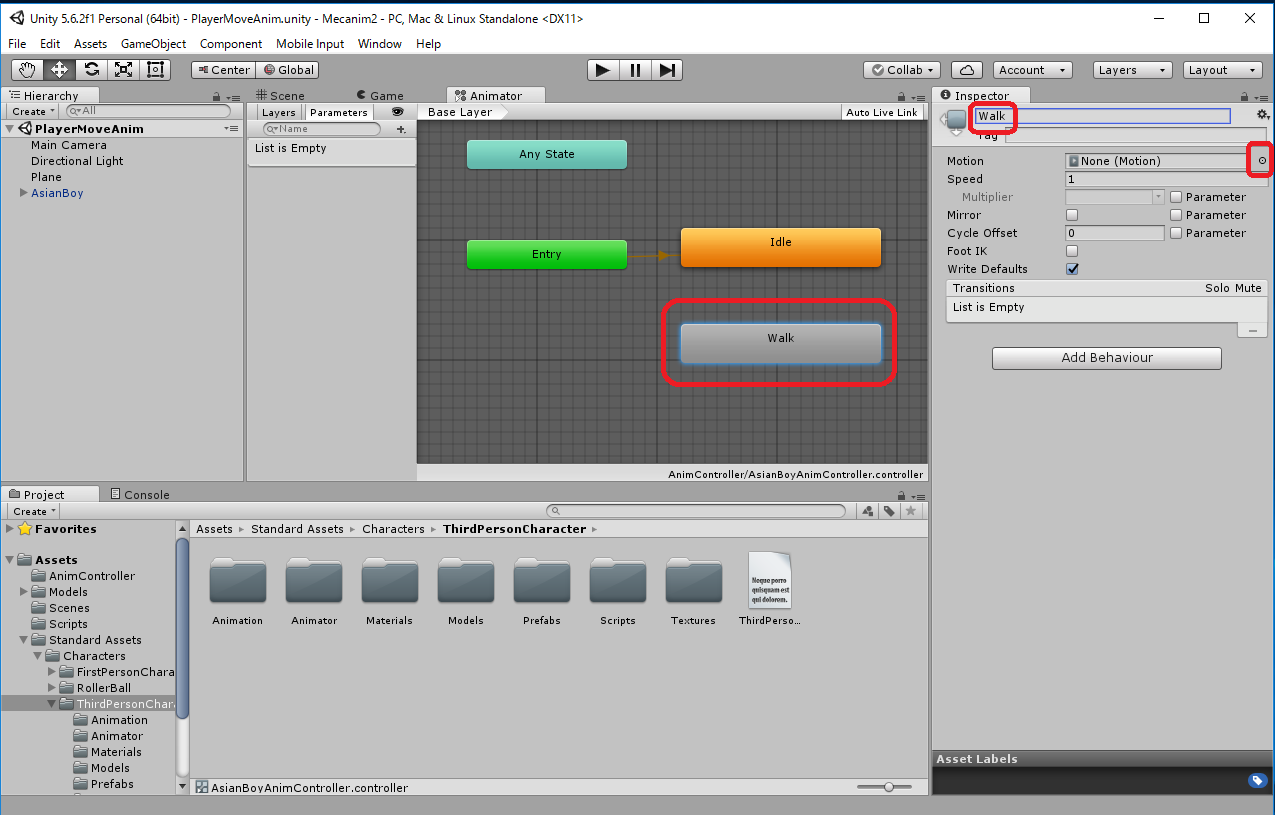
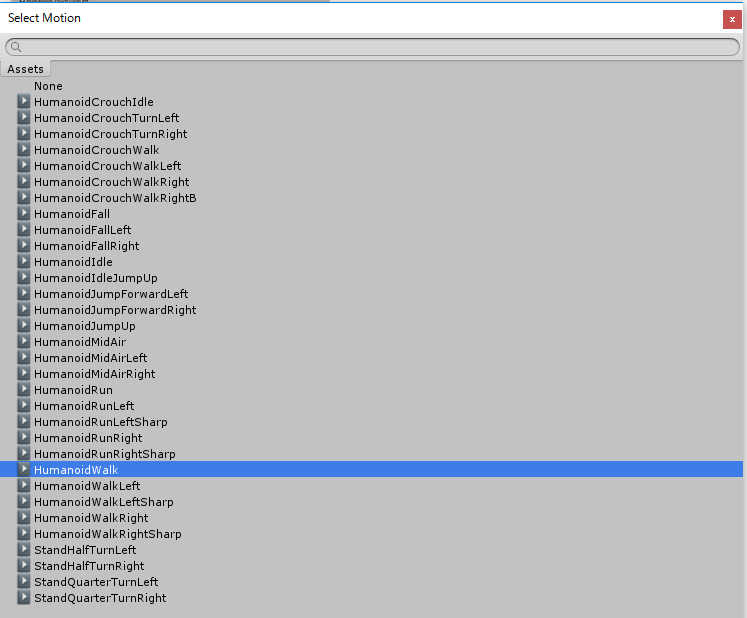
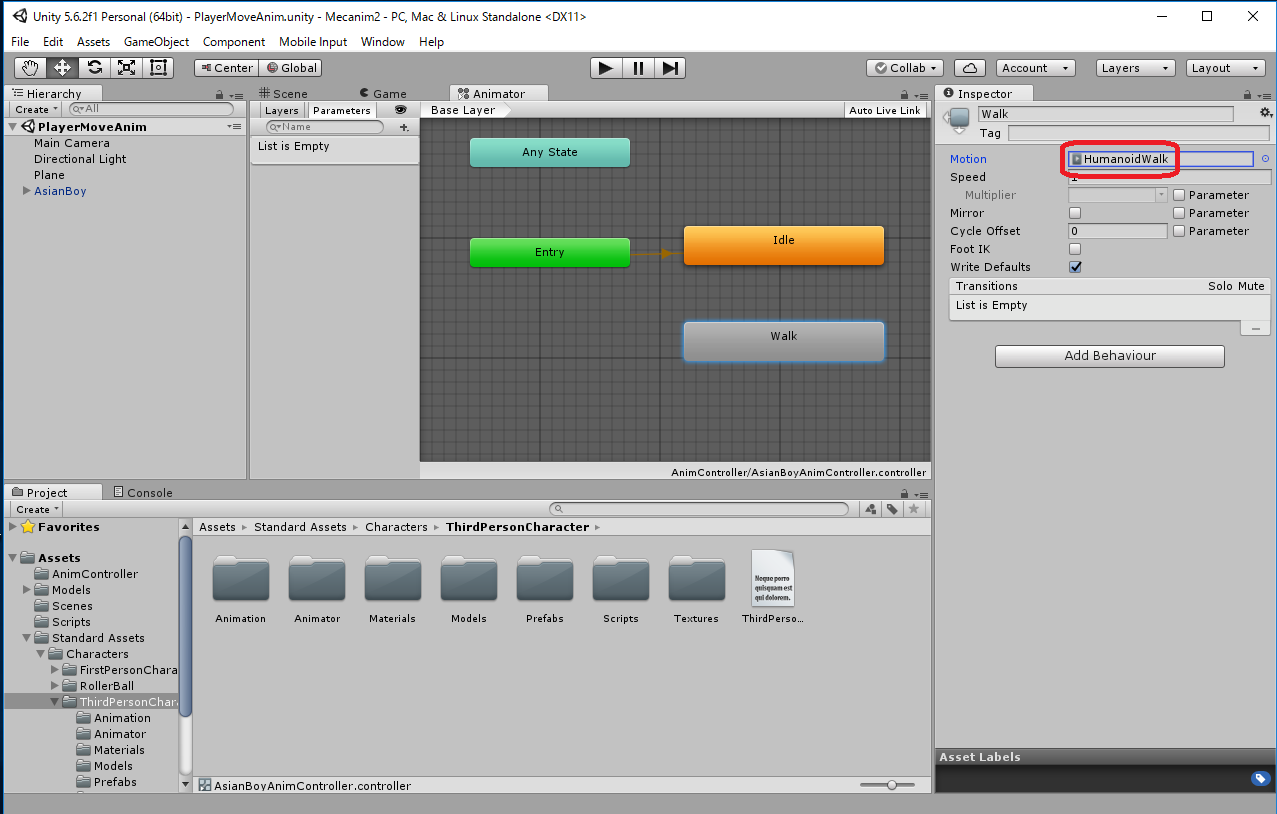
- In the Animator window, define the State Transition from "Idle" state to "Walk" state.
Right click in the Animator window -> Make Transition ->
Drag on to the "Walk"
-> an arrow from "Idel" state to "Walk" is created.
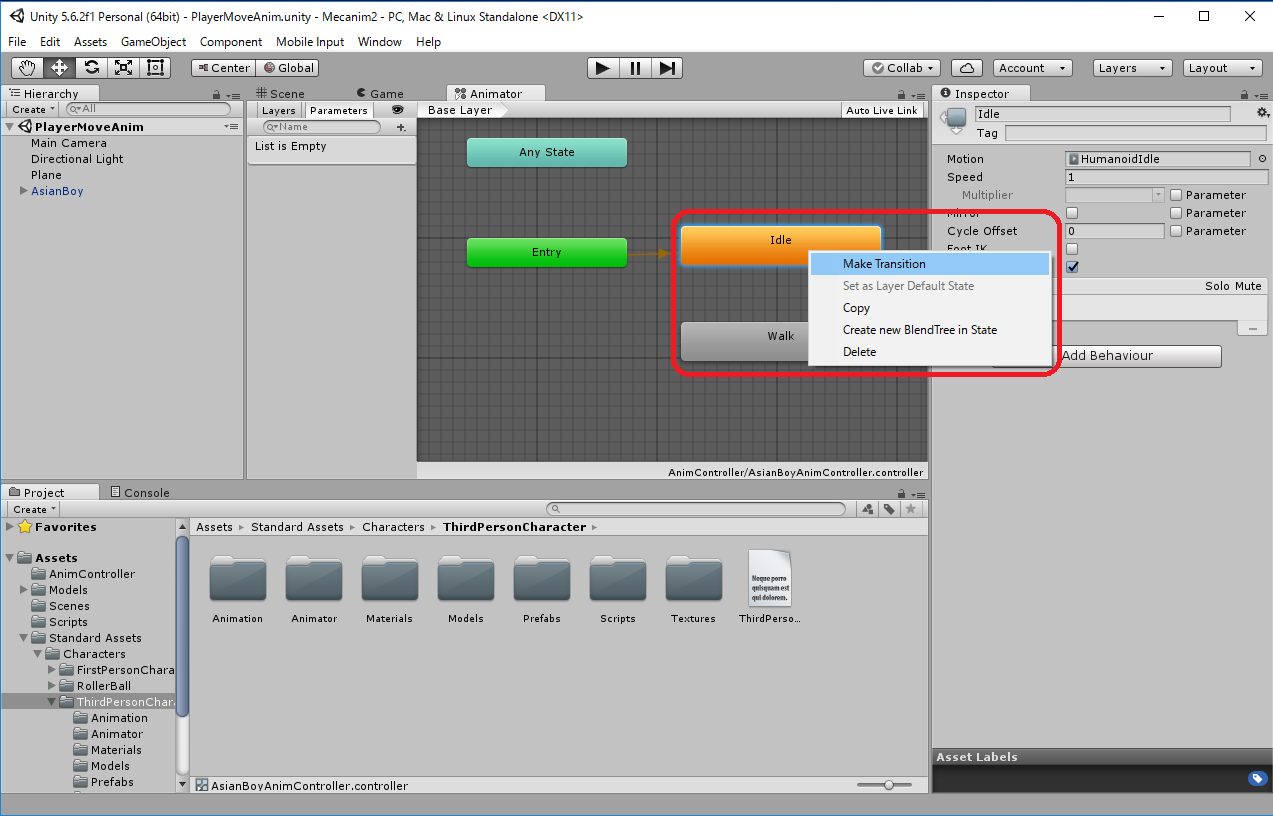
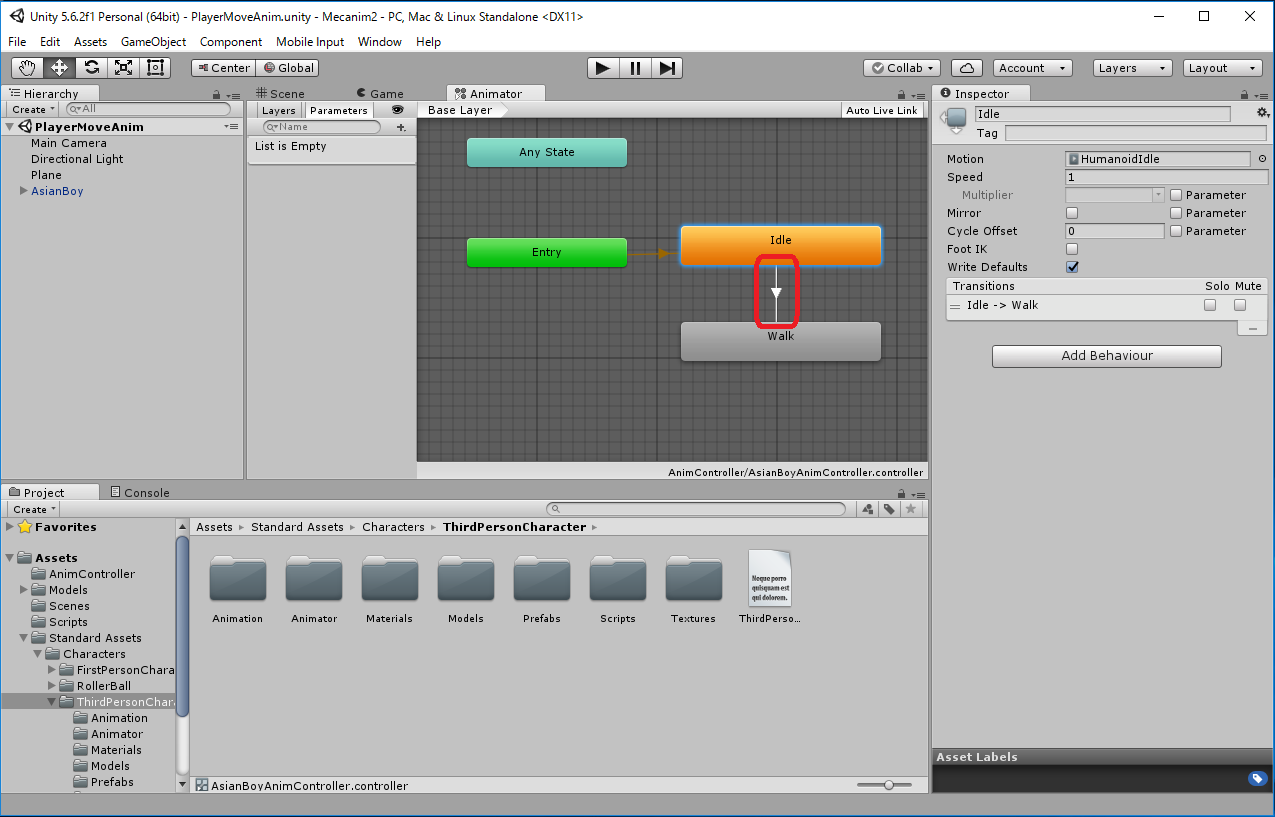
- Create a condition variable "MovingSpeed" to make a transition from "Idle" state to "Walk" state.
From the "Parameter" in the Animator window, click "+",
choose "Float",
and type the name "MovingSpeed".
The default value is 0.0 and it is ok for the initial value.
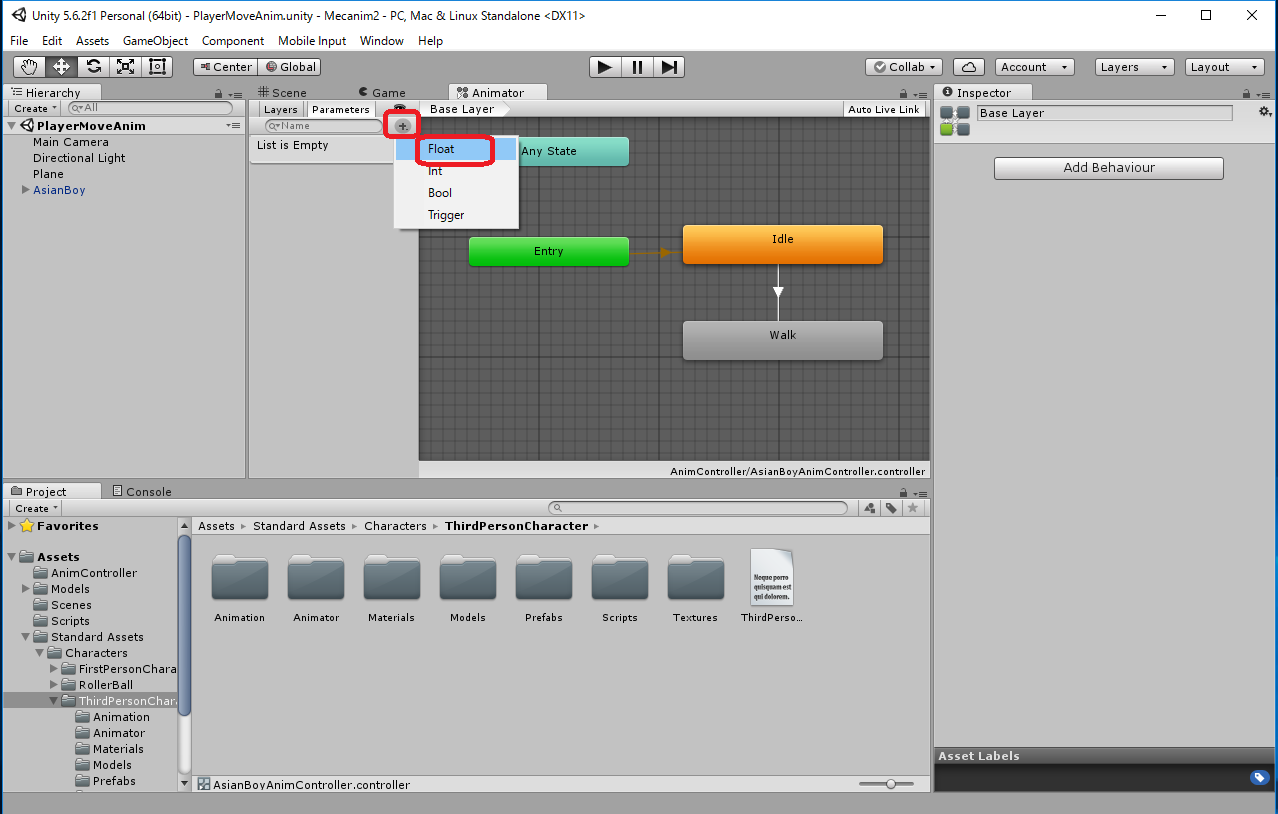
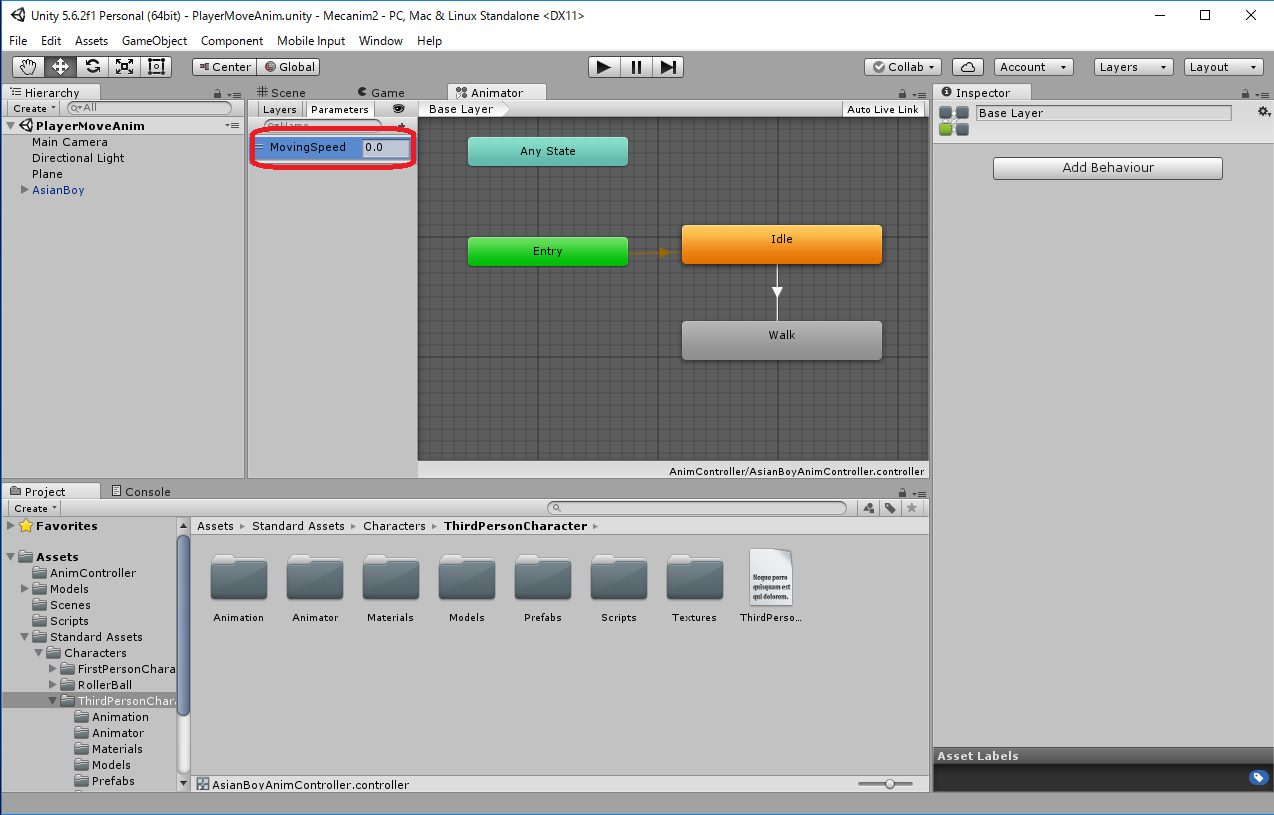
- Select the transition arrow from the "Idle" state to "Walk" state in the Animator window
by left clicking, and click "+" in the "Conditions" in the Inspector window.
Specify the transition conditions as "MovingSpeed", "Greater", "0.1".
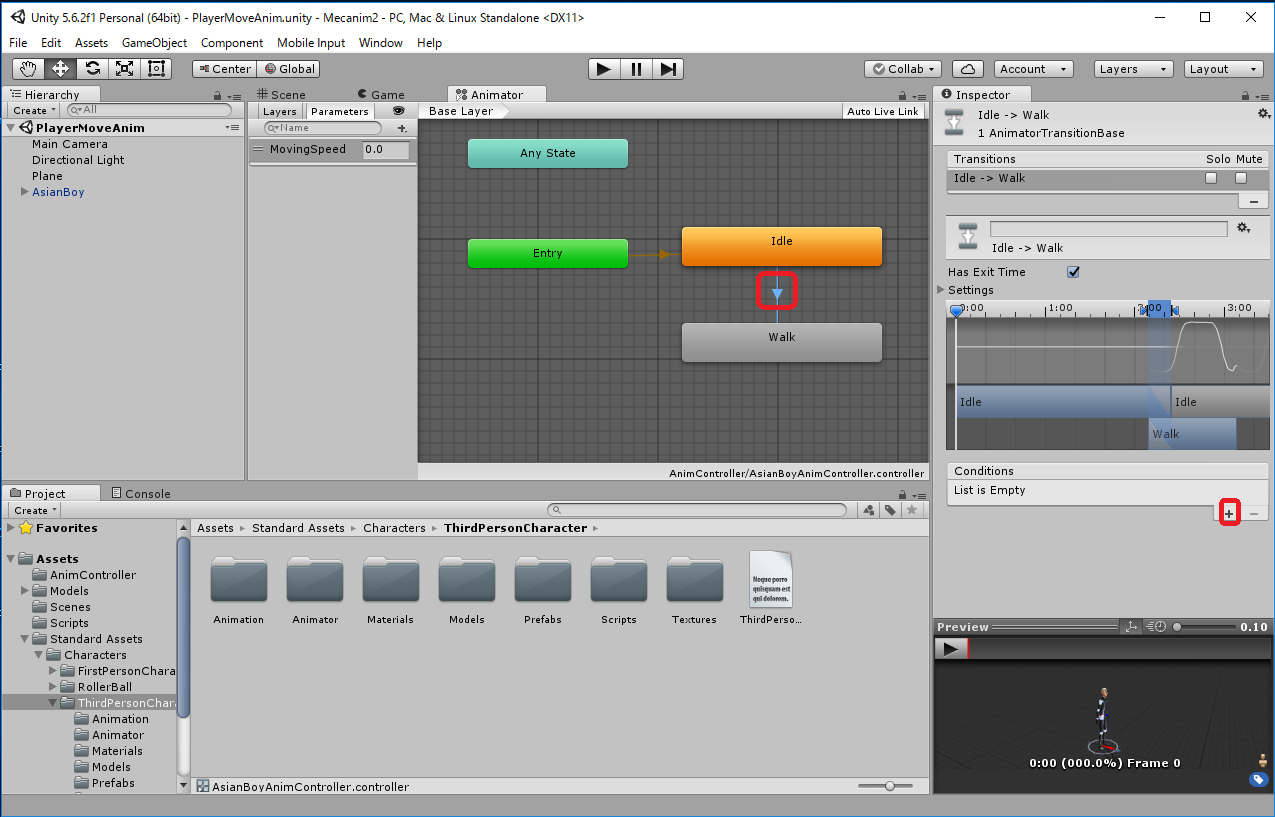
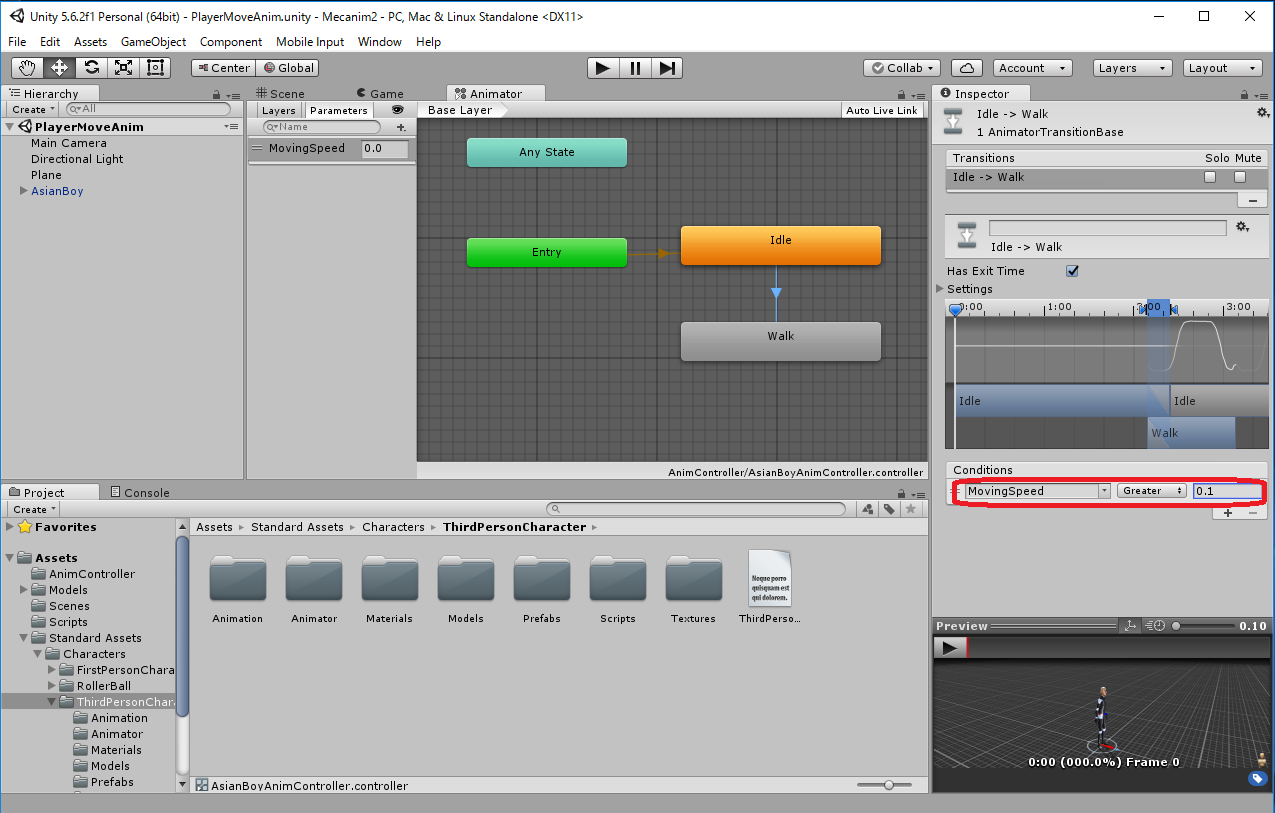
- Define the state transition from "Walk" state to "Idle" state.
Select the transition arrow from the "Walk" state to "Idle" state in the Animator window
by left clicking, and click "+" in the "Conditions" in the Inspector window.
Specify the transition conditions as "MovingSpeed", "Less", "0.1".
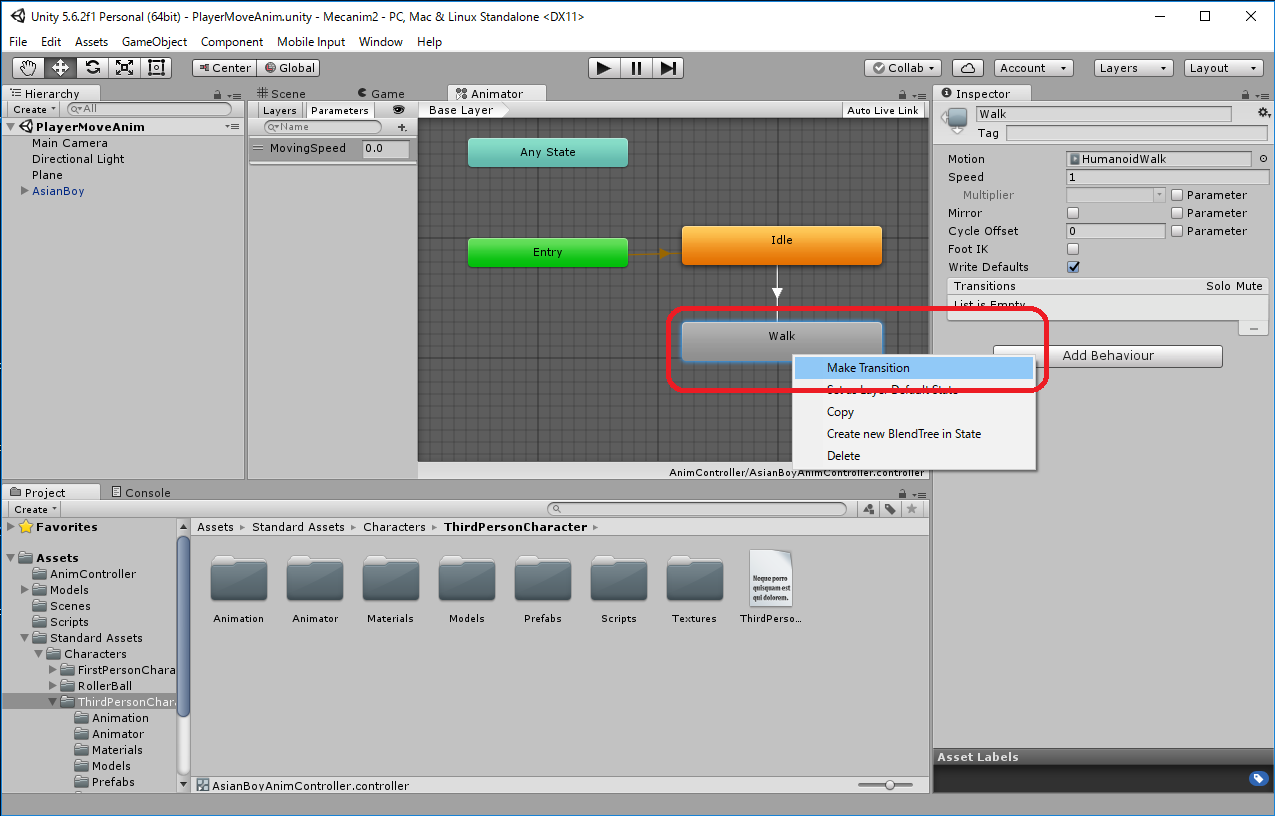
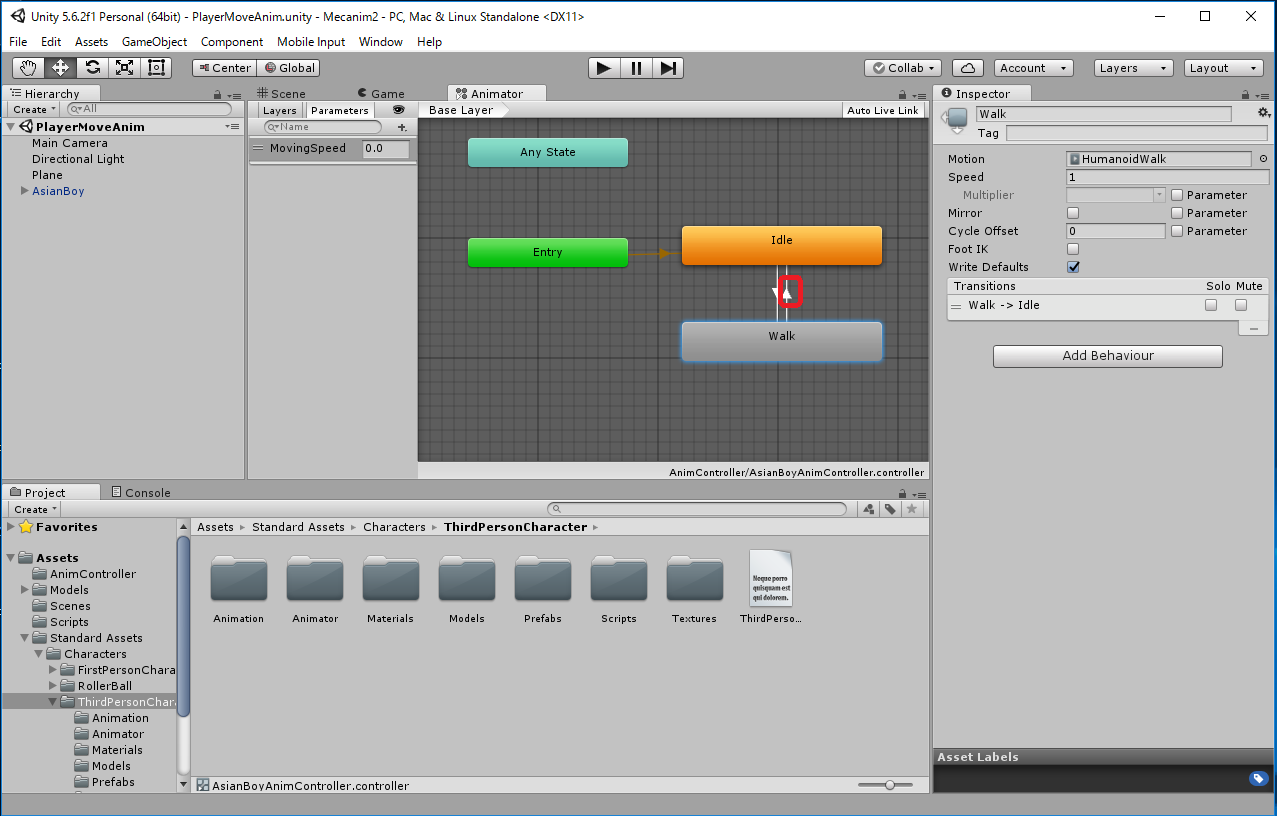
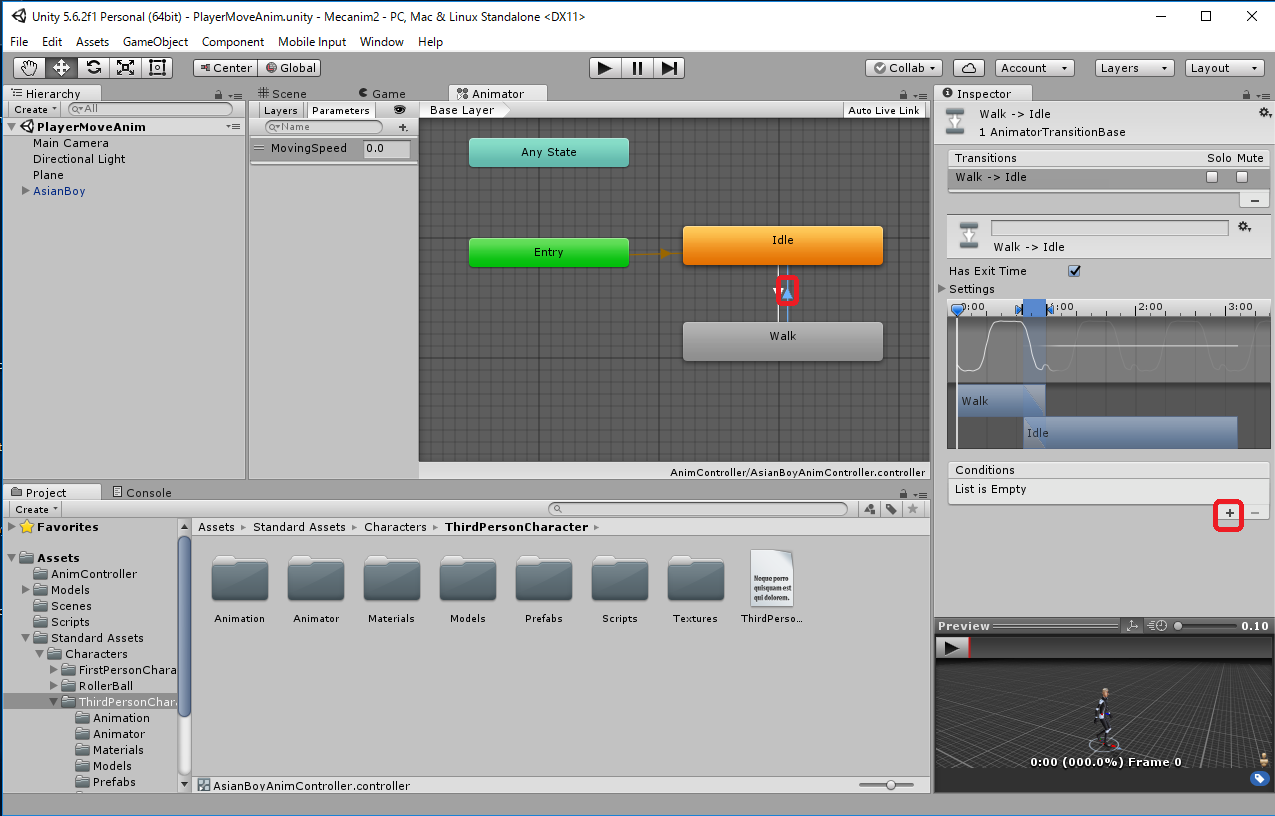
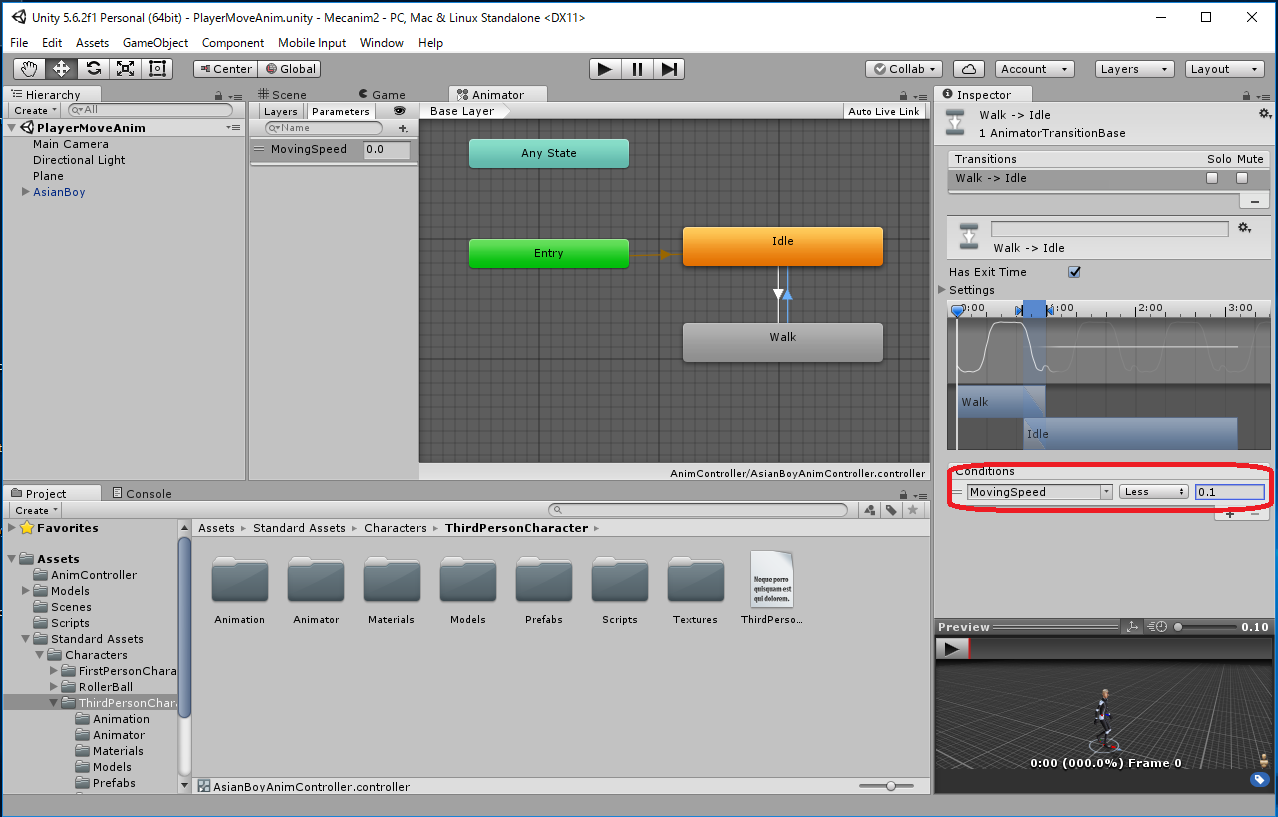
-
Select "AsianBoy" in the Hierarchy window,
and drag "PlayerAnimController" from "Assets/AnimController/" in the project window
to the "Controller" of Animator in the Inspector window.
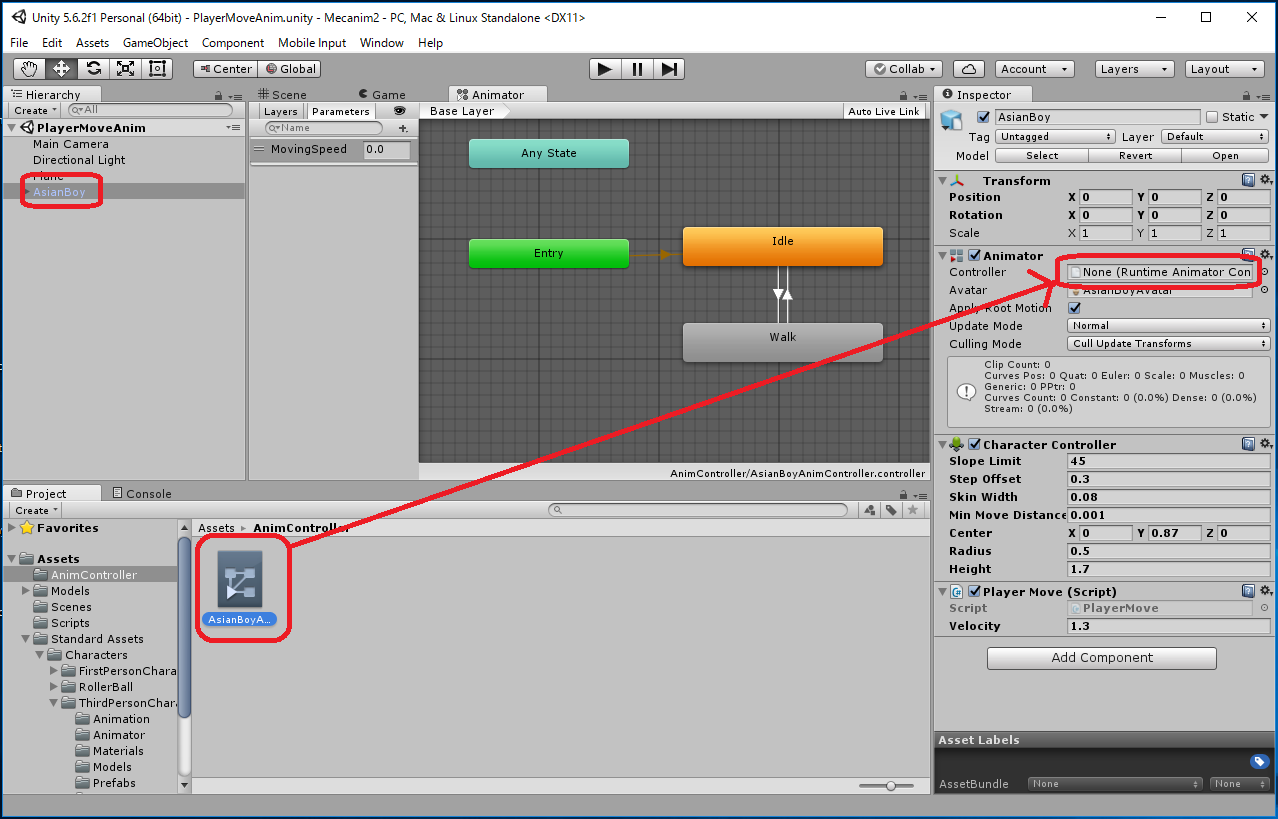
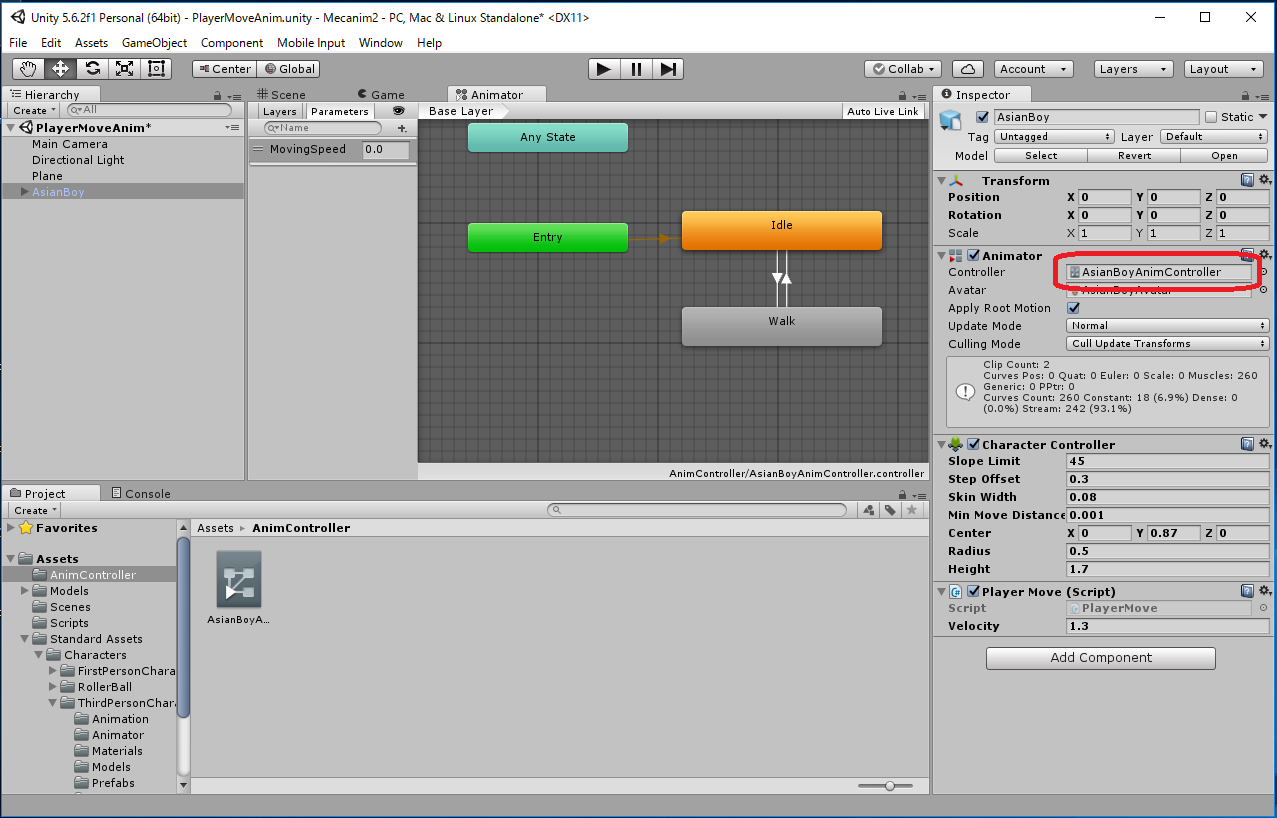
- Create C# Script "Assets/Scripts/PlayerMoveAnim.cs" and change the contents as follows.
Right click in the Assets/Scenes -> Create -> C# Script -> rename to "PlayerMoveAnim"
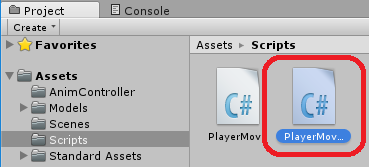
PlayerMoveAnim.cs |
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class PlayerMoveAnim : MonoBehaviour {
public float velocity = 1.3f;
private CharacterController charController;
private Animator animator;
void Start () {
charController = gameObject.GetComponent<CharacterController>();
animator = gameObject.GetComponent<Animator>();
}
void Update () {
float h = Input.GetAxis("Horizontal");
float v = Input.GetAxis("Vertical");
Vector3 moveDirection = new Vector3(0, 0, 0);;
if (charController.isGrounded) {
moveDirection = new Vector3(h, 0, v);
}
float MovingSpeed = velocity * moveDirection.magnitude;
if (MovingSpeed > 0.1f) {
animator.SetFloat("MovingSpeed", MovingSpeed);
} else {
animator.SetFloat("MovingSpeed", 0.0f);
}
transform.LookAt(transform.position + moveDirection);
moveDirection.y += Physics.gravity.y;
charController.Move(velocity * Time.deltaTime * moveDirection);
}
}
|
-
Drag "Assets/Scripts/PlayerMoveAnim" in the Project window onto the "AsianBoy" in the Hierarchy window,
then the "Player Move Anim (Script)" component is added to the "AsianBoy".
Remove the check of the "Player Move (Script)" added before in the Inspector window,
because we will not use this now.
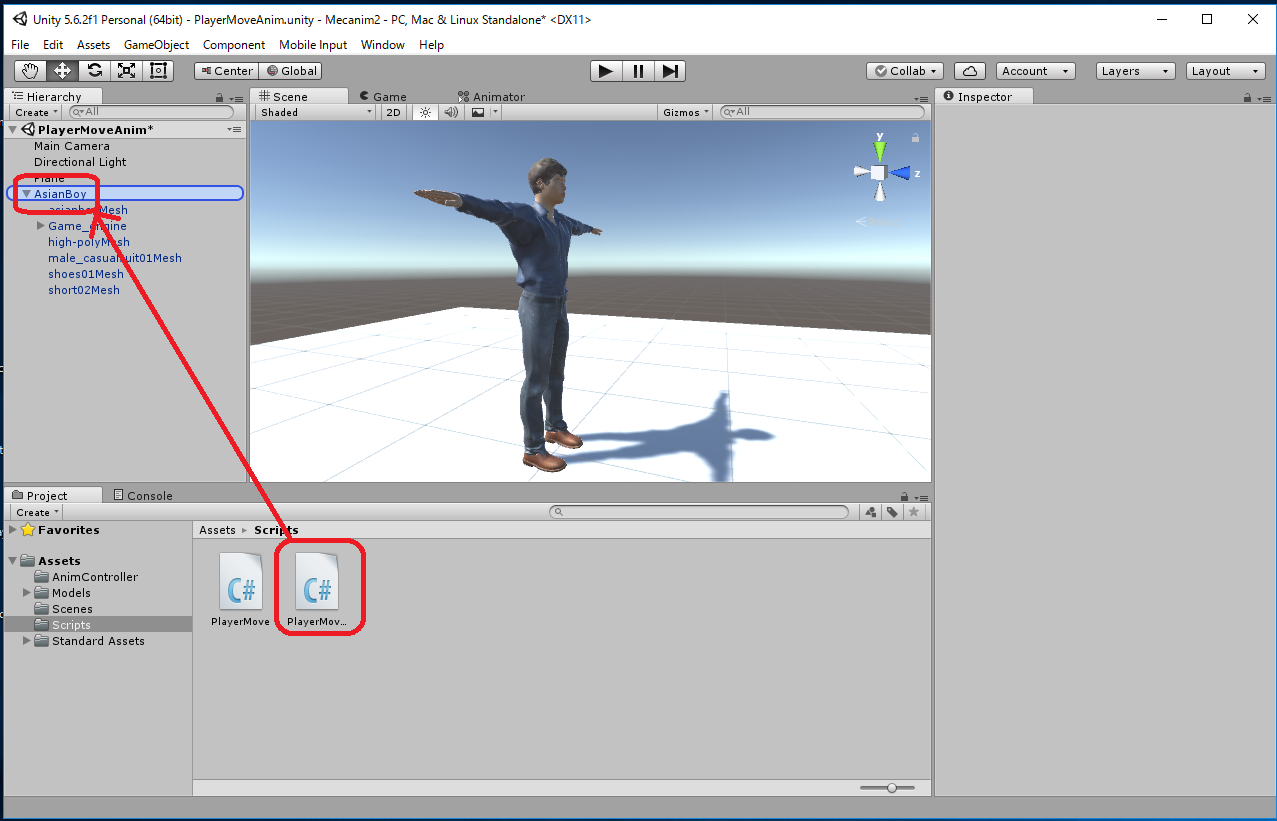
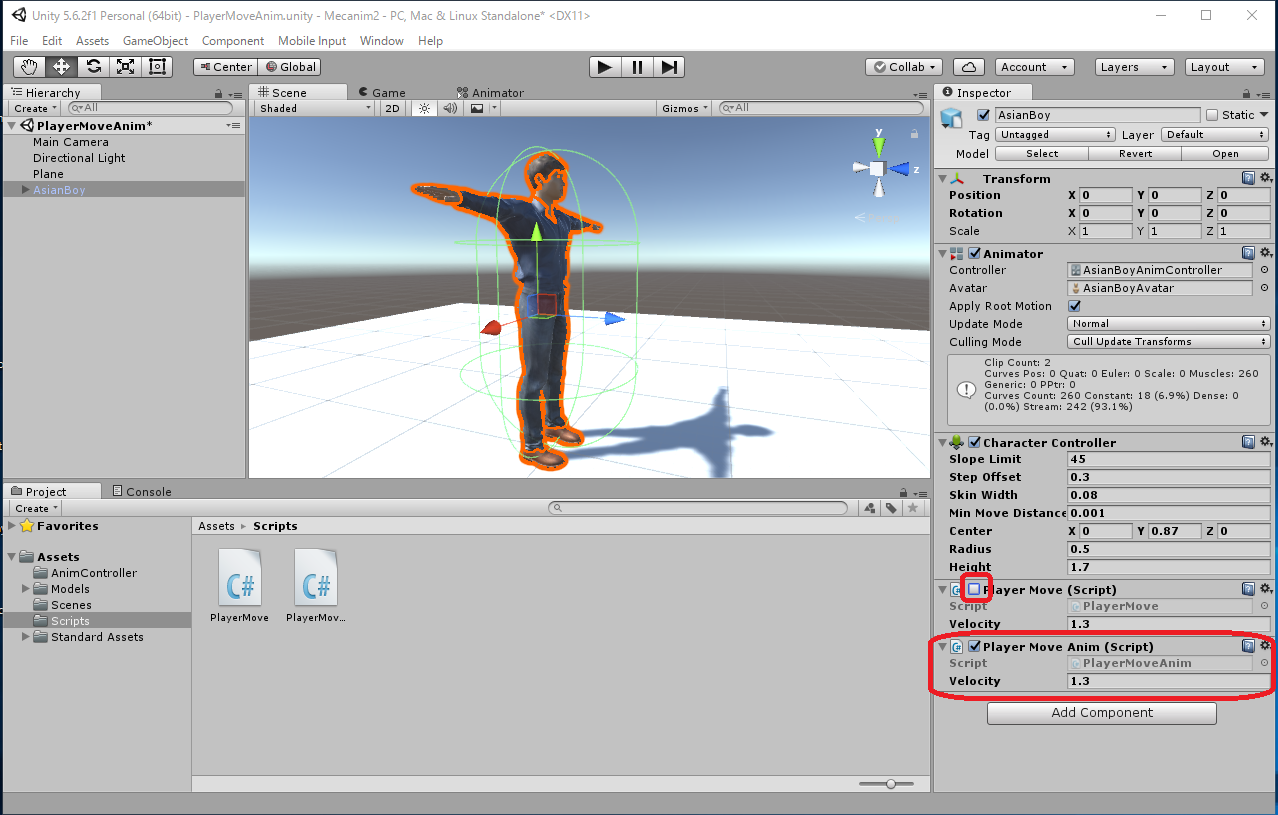
- Click "Play" button
to execute.
"AsianBoy" moves with the keyboard arrow keys (↑, ↓, ←, →)
or the 'w', 'a', 's', 'd' keys.
You can see that animation of the Humanoid Character switches according to the state.
However, switching of behavior seems to be somewhat slow.
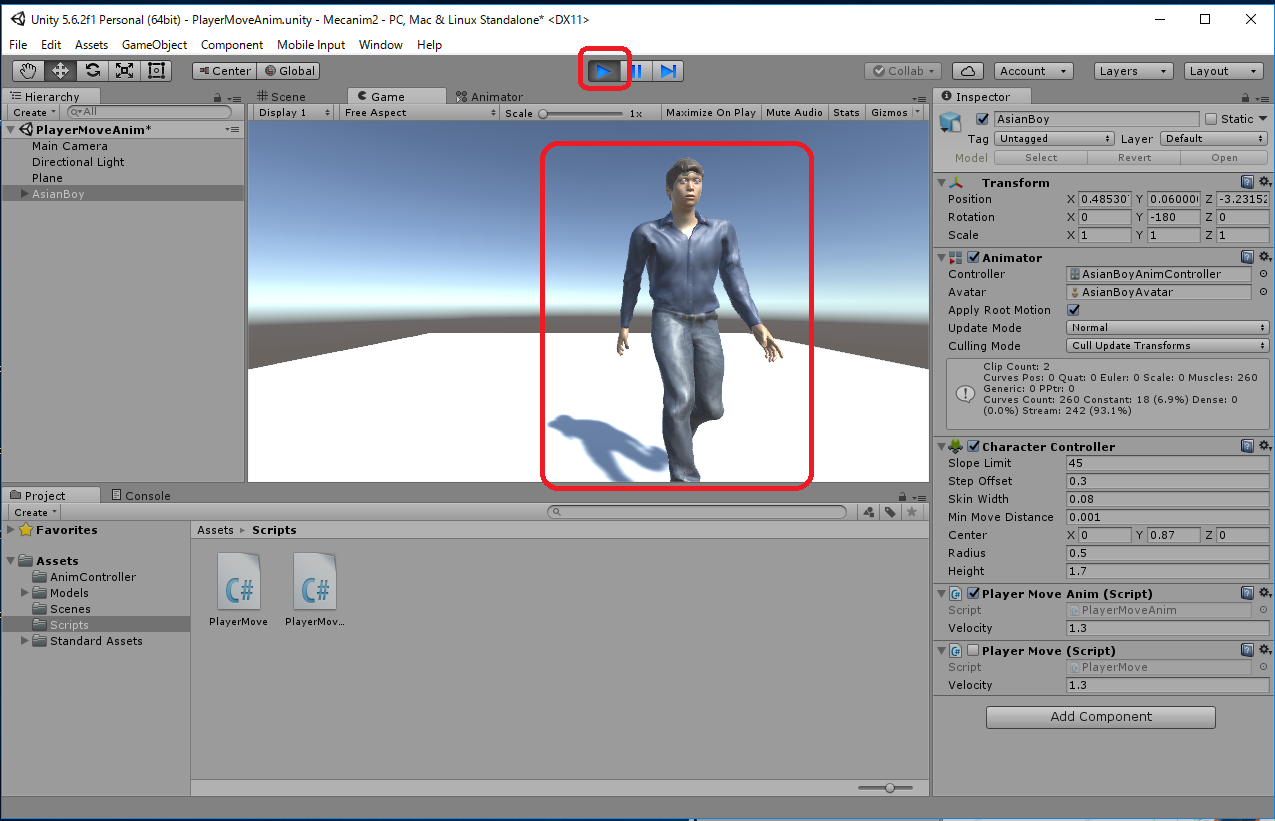
-
In the Animator window, select "State transition from Idle state to Walk state"
and "State transition from Walk state to Idle state", and uncheck "Has Exit Time"
in the Inspector window.
If this is unchecked, it will take as much time as usual to state transition.
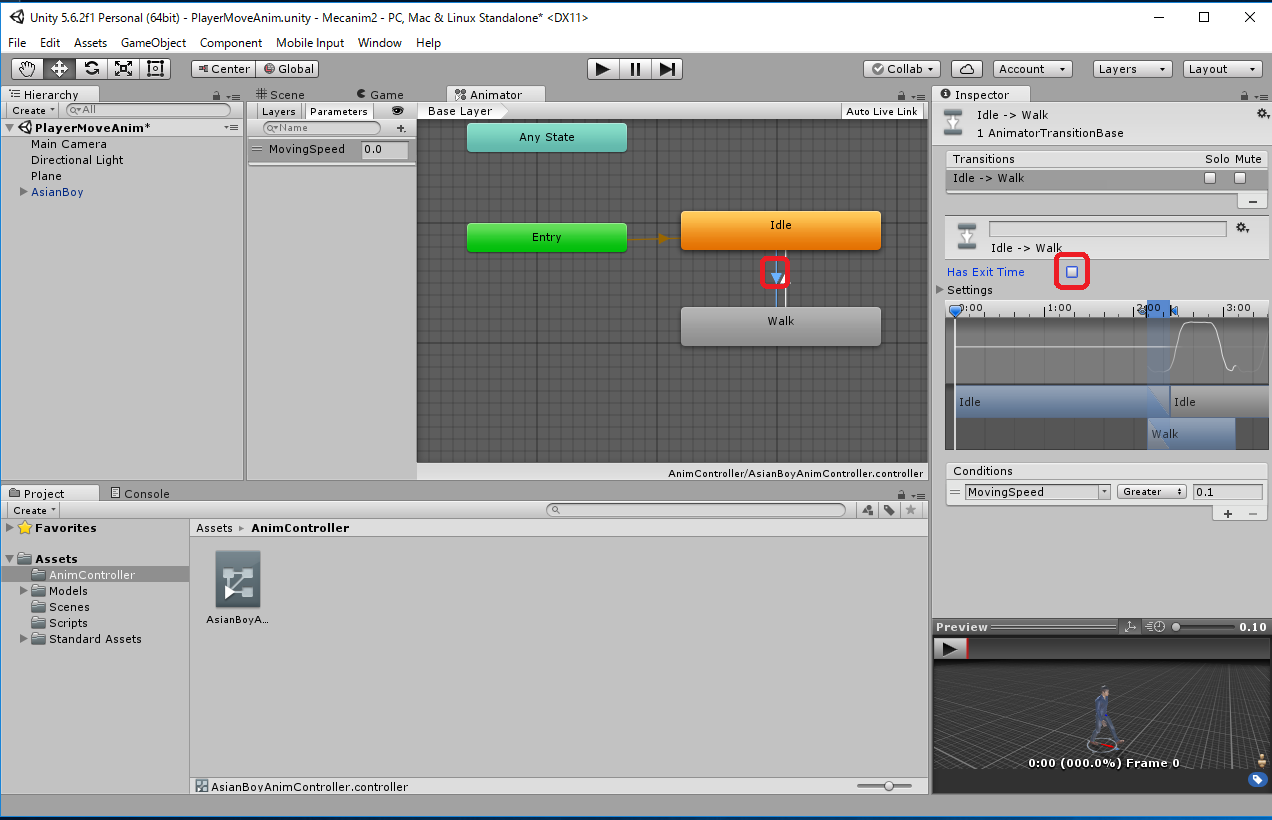
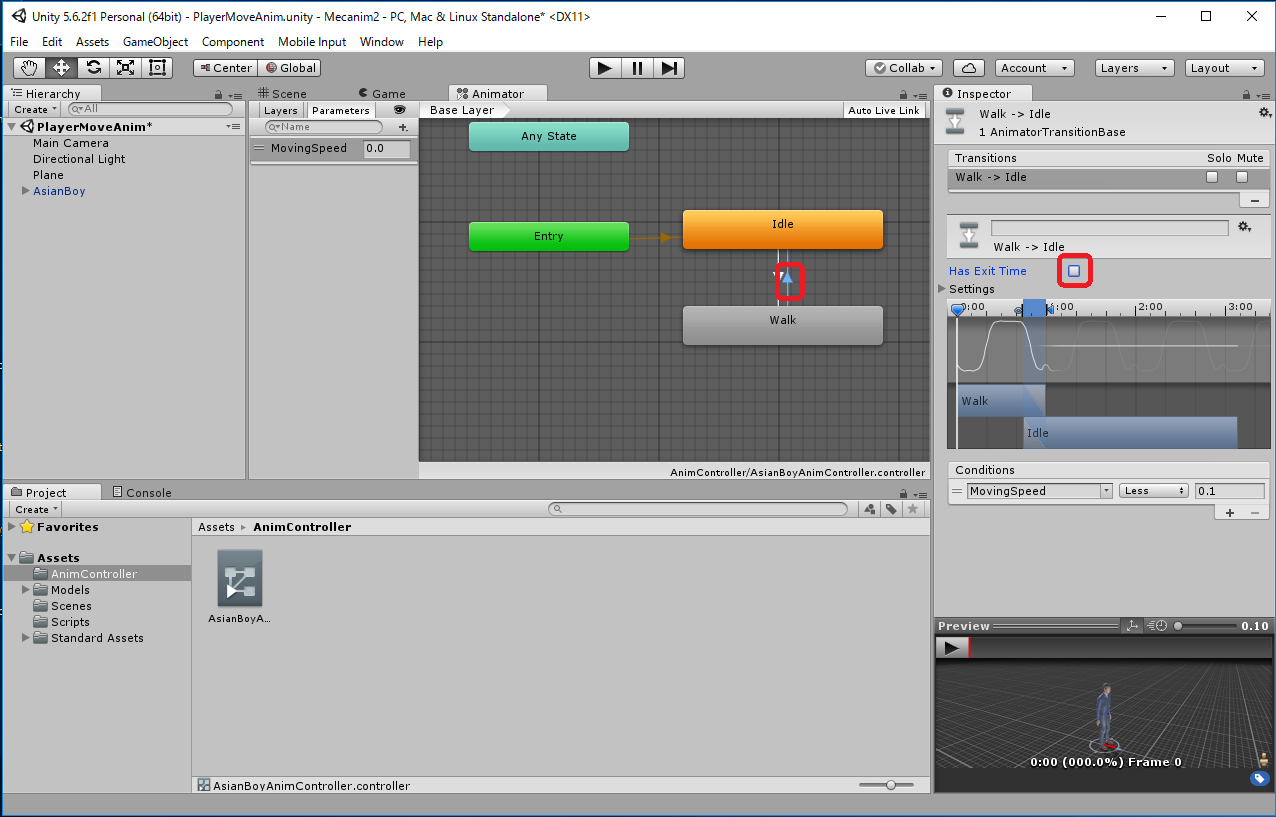
- Save Scene.
We already created the "PlayerMoveAnim.unity" scene, so overwrite it.
File -> Save Scenes
-
Here is the Unity's project file Mecanim2.zip I explained here。