- Start a new project with Unity.
Start a new project named "Mecanim" of "3D" type.
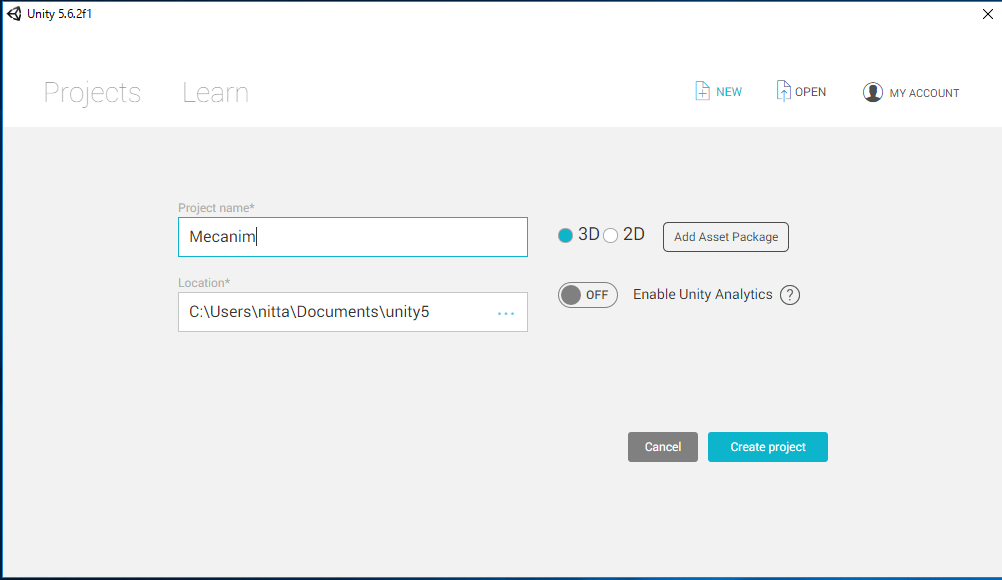
- Import your Humanoid model (fbx) into Unity.
- Create a folder named "Models" in the Asset.
Assets -> Create -> Folder -> rename as "Models"
- In Explorer, open the "Documents/makehuman/v1/exports/" folder created by MakeHuman.
From there, import "AsianBoy.fbx" and "textures/" folder by dragging
to "Assets/Models/" folder in Unity's project window.
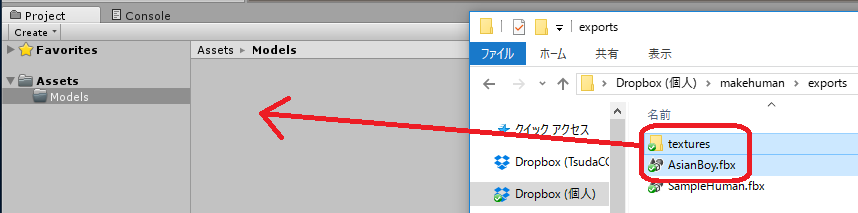
[Notice]
The above operation may be done from the menu,
Assets -> Import New Asset... -> AsianBody.fbx
,
but at this time the texture required for AsianBoy.fbx will not be imported
automatically and the model will be completely white.
In this case, you have to import hte texture correspond to the white material generated in
"Assets/Models/Materials/" manually.
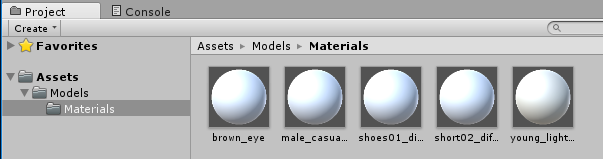
- In the project window, select "AsianBoy".
In the "Rig" tab of inspector window,
change the Animation Type to "Humanoid",
leave Avatar Definition as "Create From This Model" and click the "Apply" button.
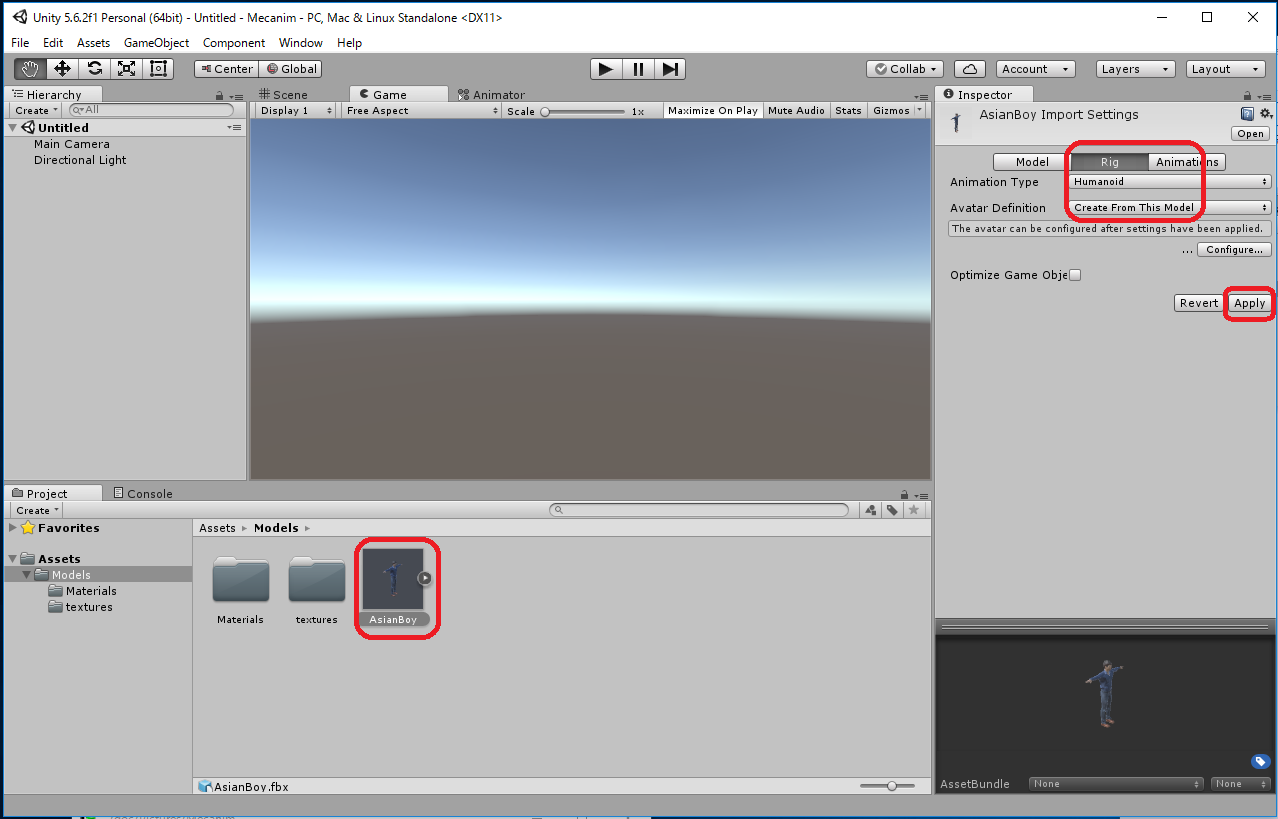
- It is better to click "Configure" in the inspector window
and make sure that the bone is set correctly.
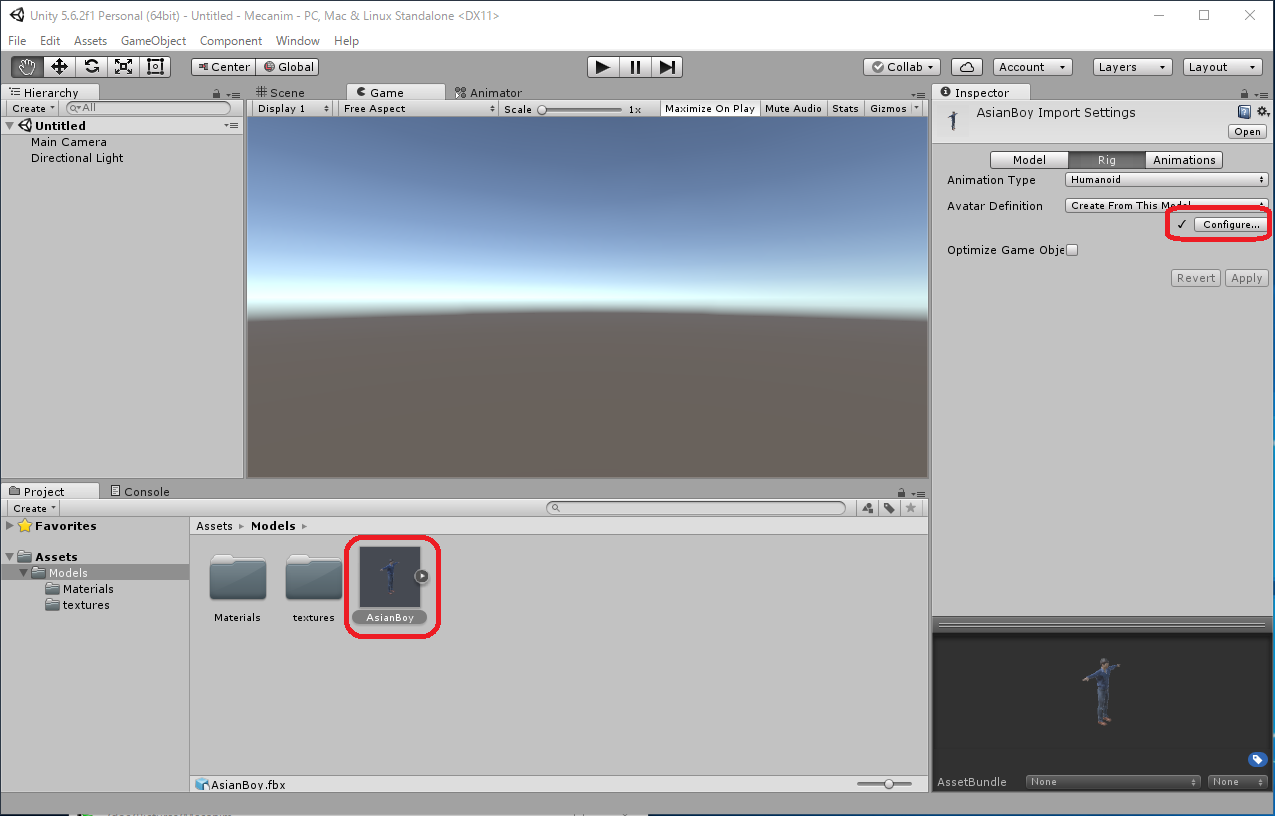
- Click "Mapping" tab in the Inspector window to see how correspondence of bones.
The display may be better for the Scene window than for the Game window.
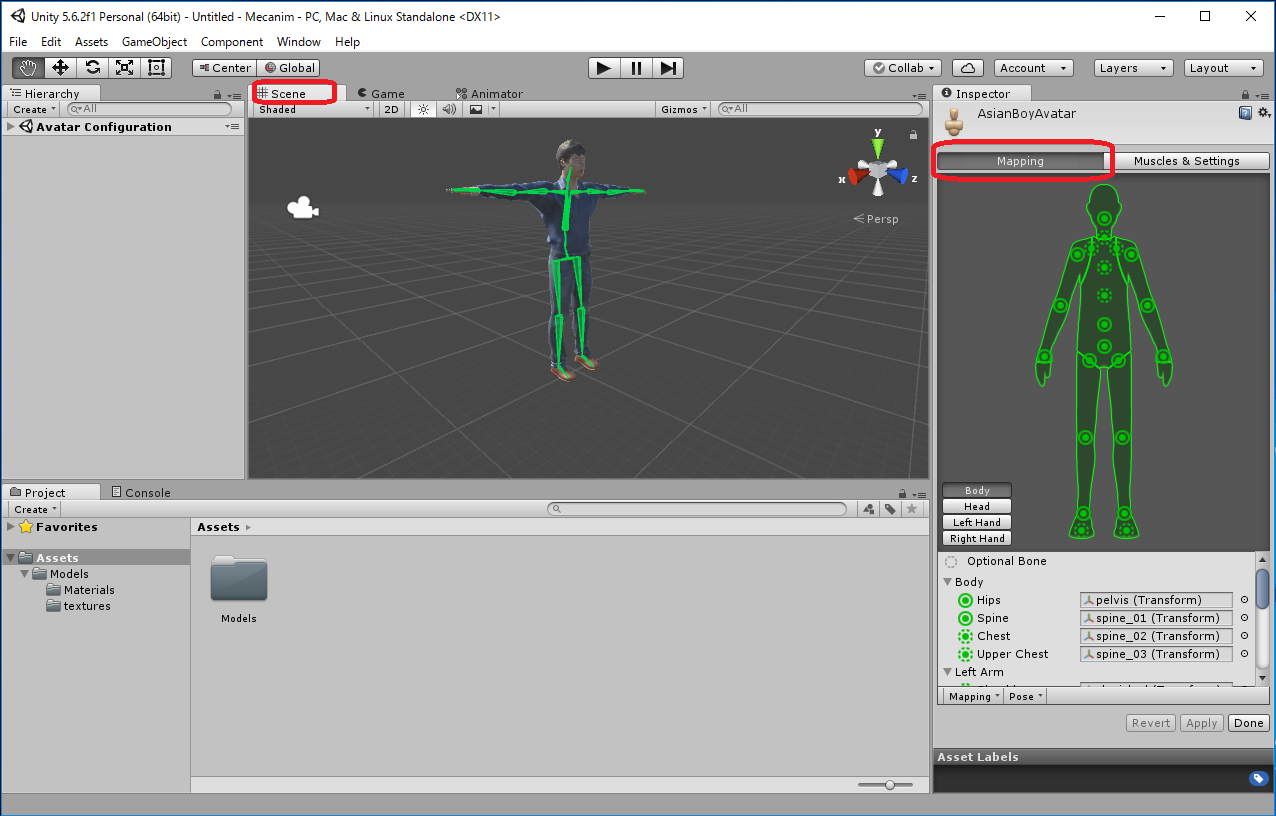
- In "Muscles & Settings" tab of the inspector window,
move the sliders to see if each bone works properly.
When you can confirm, click "Done".
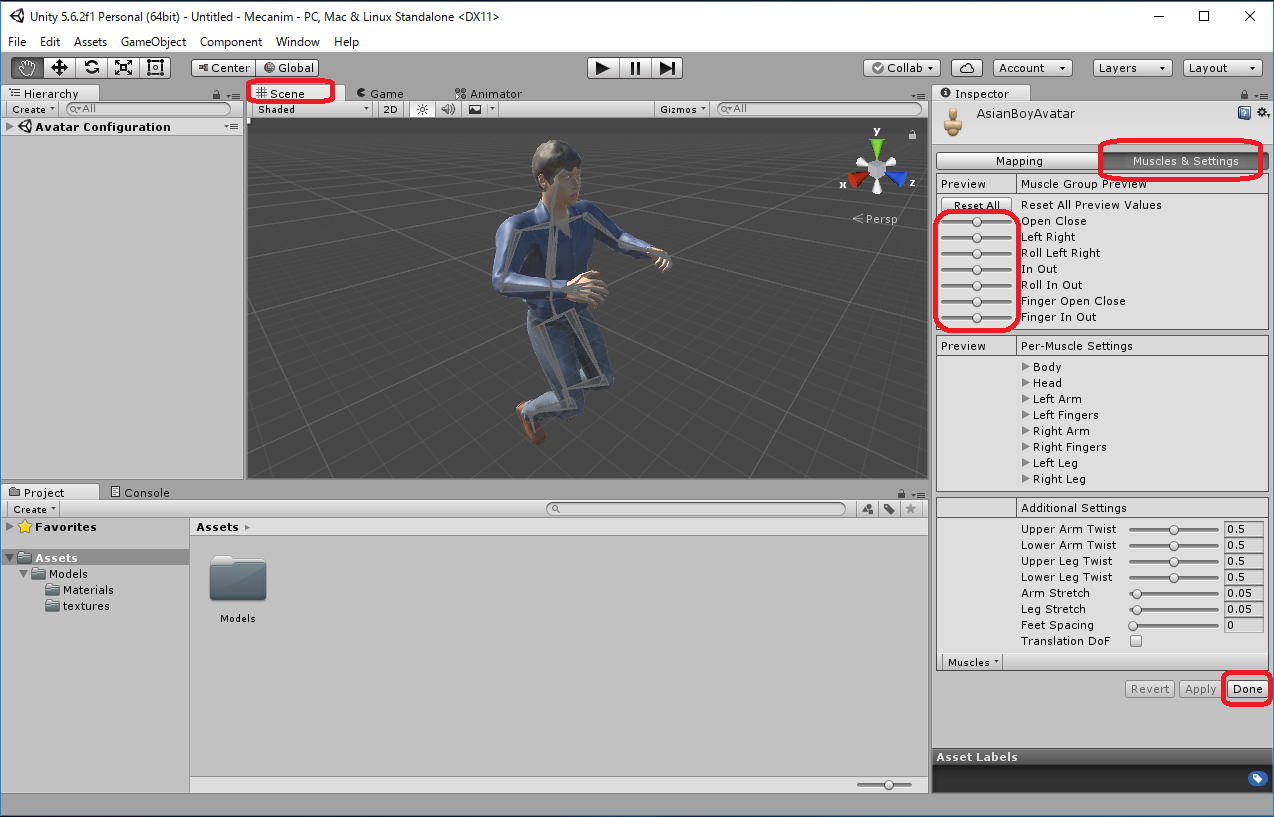
- Create a Plane as a ground in the Hierarchy.
Choose Reset from Settings
of Transform in the Inspector window
to set Position (x,y,z)=(0,0,0).
GameObject -> 3D Object -> Plane
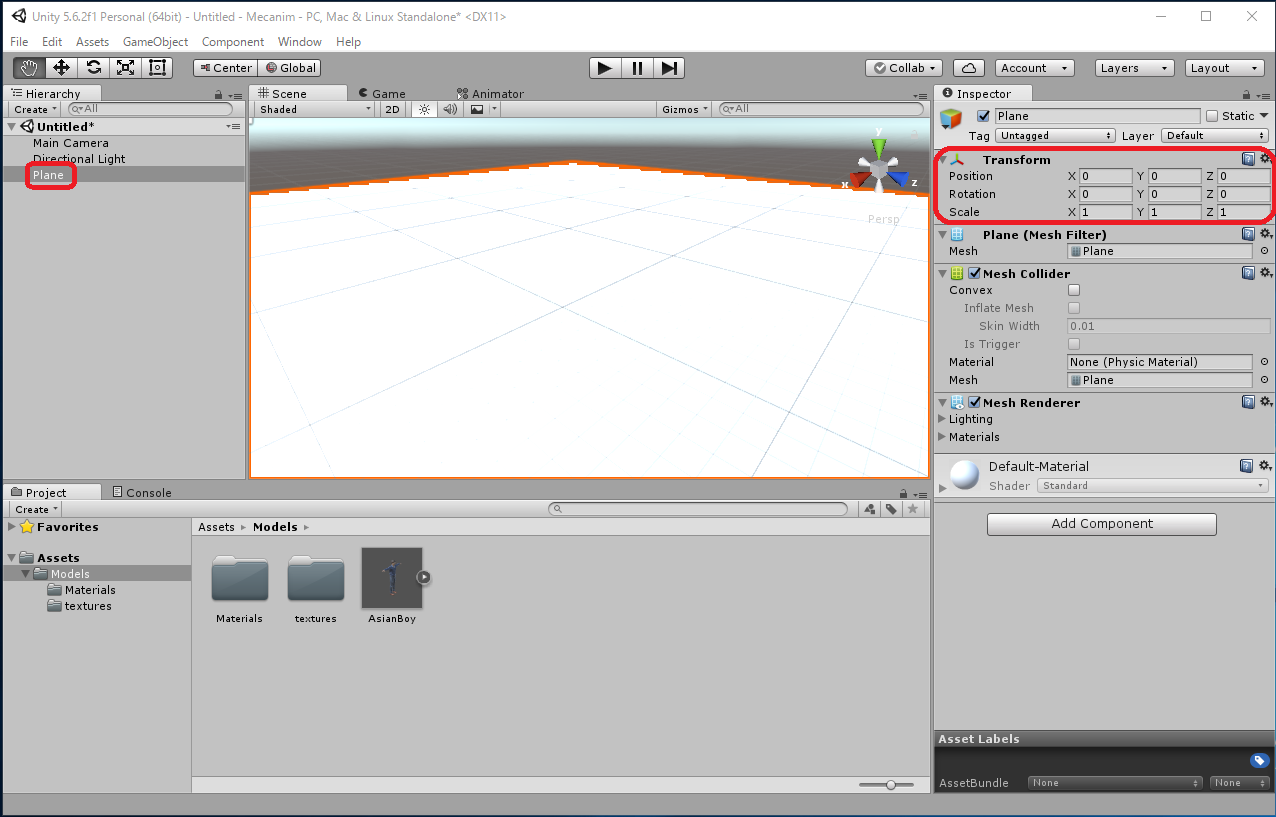
- Insert "fAssets/Models/AsianBoy" into the Herarchy window.
- Drag "fAsianBoy" from "Assets/Models" of the Project window into Hierarchy window.
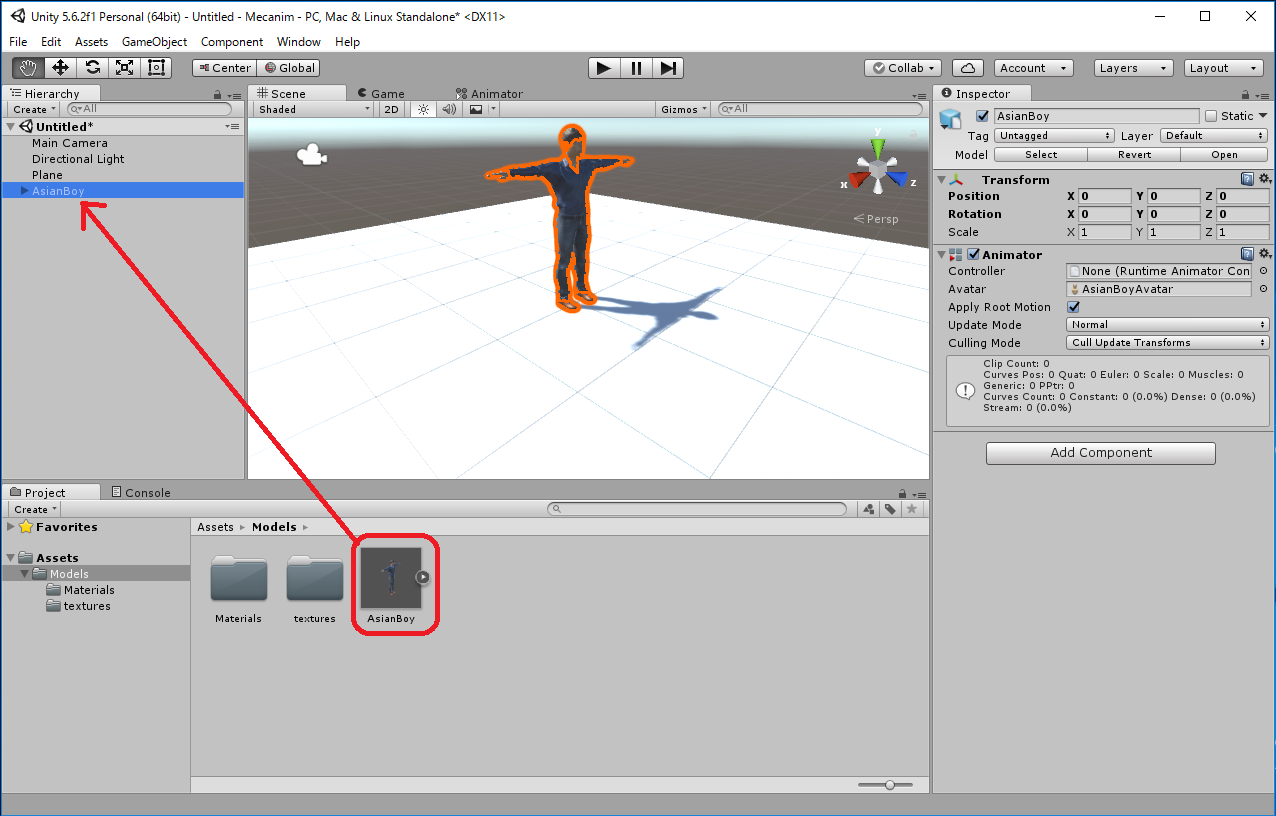
- Choose "Reset" from the Settings
of Transform in the Inspector window,
set Position (x,y,z)=(0,0,0).
- Click "Add Componenct" button in the Inspector window, and
add "Physics/CharacterController" component.
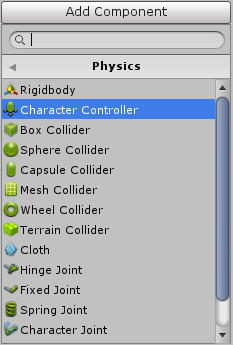
- Change the "Center" and "Height" values of "CharacterController" component
added in the Inspector window.
While viewing the Scene window, if you select "AsianBoy" in the Hierarchy,
the CharacterController's Capsel Collider will be shown with a green solid line.
Change "Center" and "Height" values to match humanoid character.
In this example, Center(x,y,z) = (0, 0, 87.0), Height = 1.7.
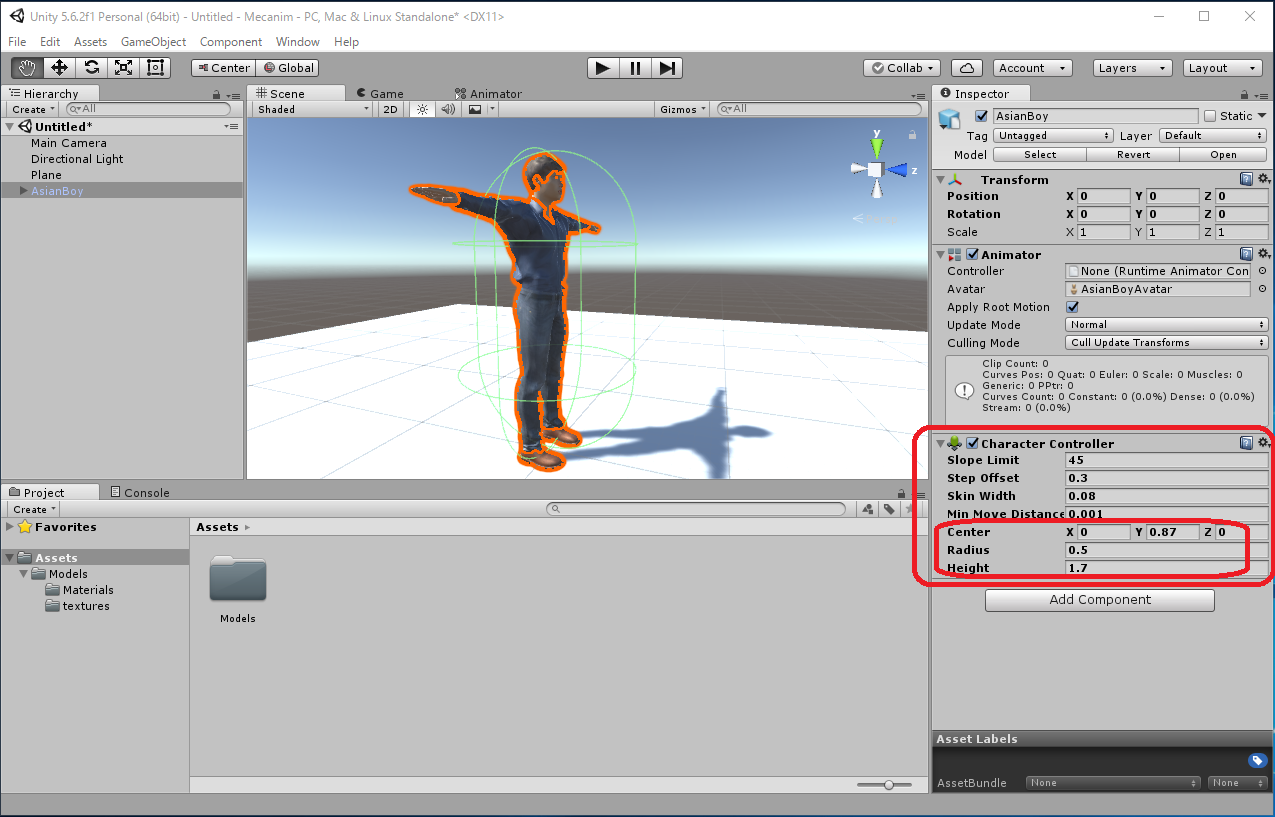
- Create a script to move the Humanoid Character.
- Create a "Scripts" folder in the Assets of Project window.
Right click in the "Assets" -> Create -> Folder -> Scripts -> rename to "Scripts"
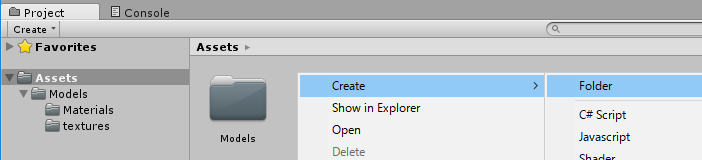
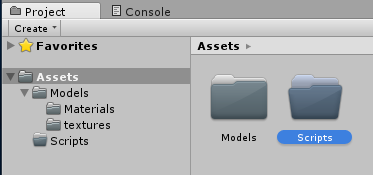
- Create a C# Scripts "PlayerMove" in the "Assets/Scripts" in the Project window.
Right click in the "Assets/Scripts" -> Create -> C# Script -> rename to "PlayerMove"
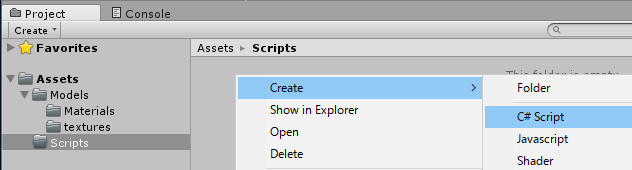
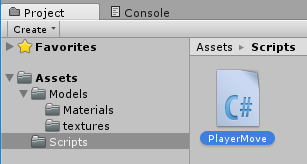
- Drag "Assets/Scripts/PlayerMove" in the Project window onto Hierarchy's "AsianBoy"
and drop it with Herarchy's "AsianBoy" surrounded by a blue ellipse.
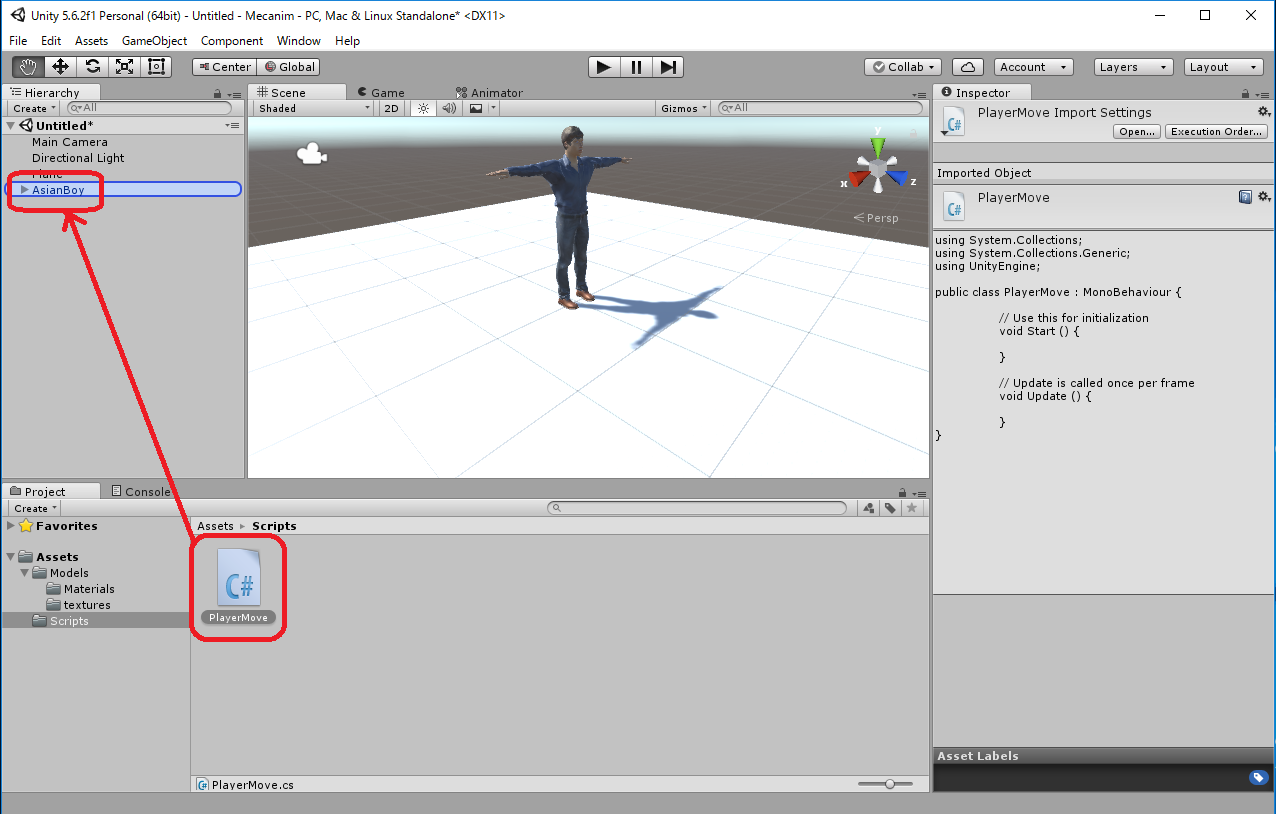
-
When you select "AsianBoy" in the Hierarchy,
"Player Move (Script)" component appears in the Inspector window,
so you can see that it was added.
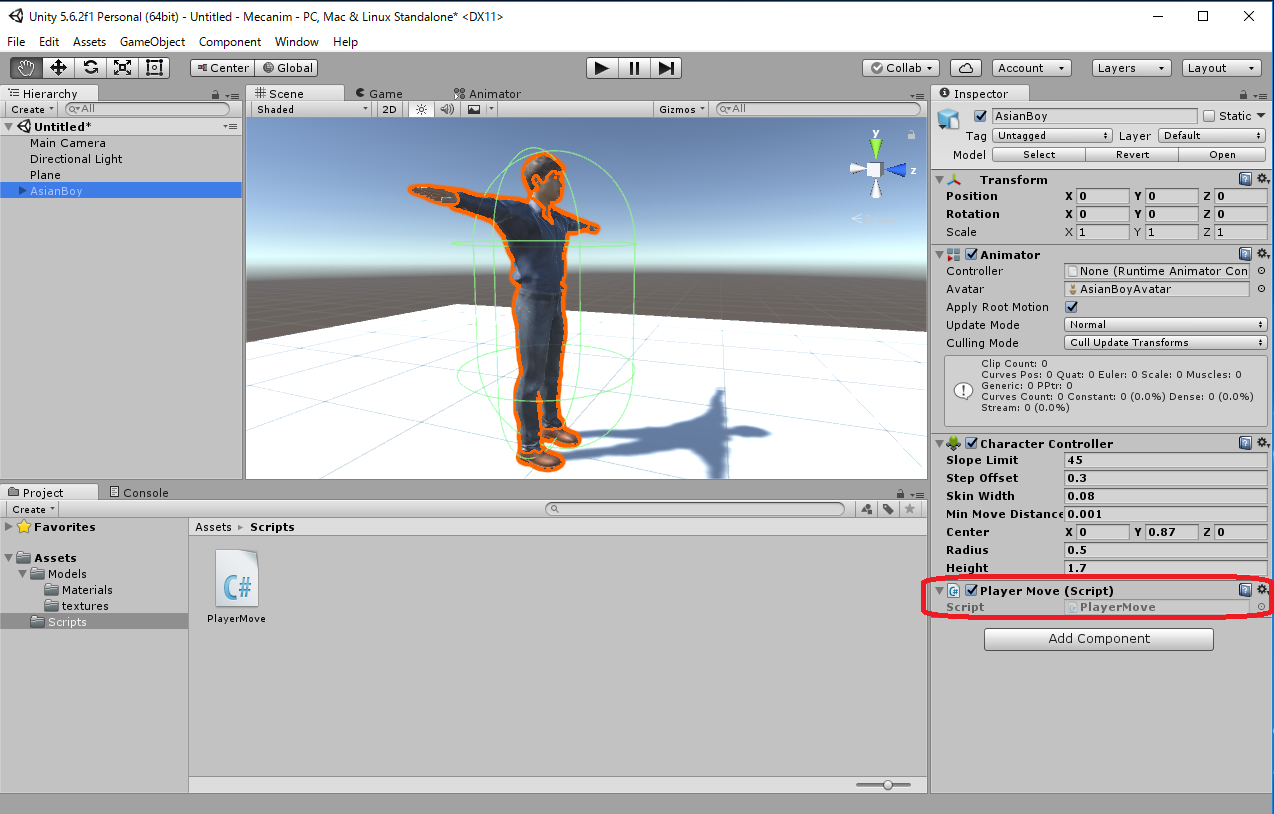
- Change the "Assets/Scripts/PlayerMove.cs" in the Project windows.
PlayerMove.cs |
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class PlayerMove : MonoBehaviour {
public float velocity = 1.3f;
private CharacterController charController;
void Start () {
charController = gameObject.GetComponent<CharacterController>();
}
void Update () {
float h = Input.GetAxis("Horizontal");
float v = Input.GetAxis("Vertical");
Vector3 moveDirection = new Vector3(h, 0, v);
charController.Move(velocity * Time.deltaTime * moveDirection);
}
}
|
- Change the Main Camera position in the Hierarchy.
Ground is a XZ-Plain whose size is 10x10 centered on the origin.
Also, "AsianBoy" is at the origin.
Let's set Transform Position (x, y, z) = (0, 1, -5) which is the end of Plane
becase the Main Camera is too far away.
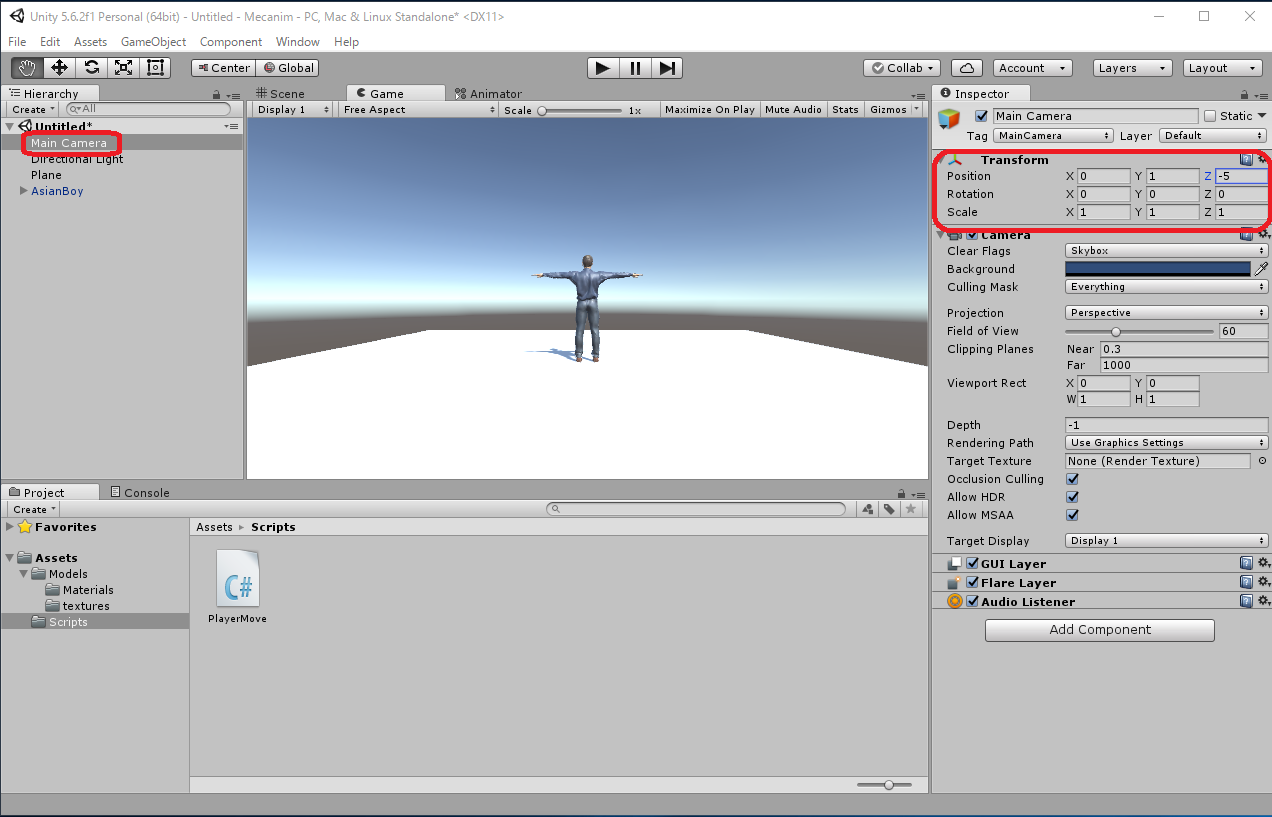
- Click the Play button
to execute.
"AsianBoy" moves with the keyboard arrow keys (↑, ↓, ←, →)
or the 'w', a', 's', and 'd' keys.
But it has floated in the air.
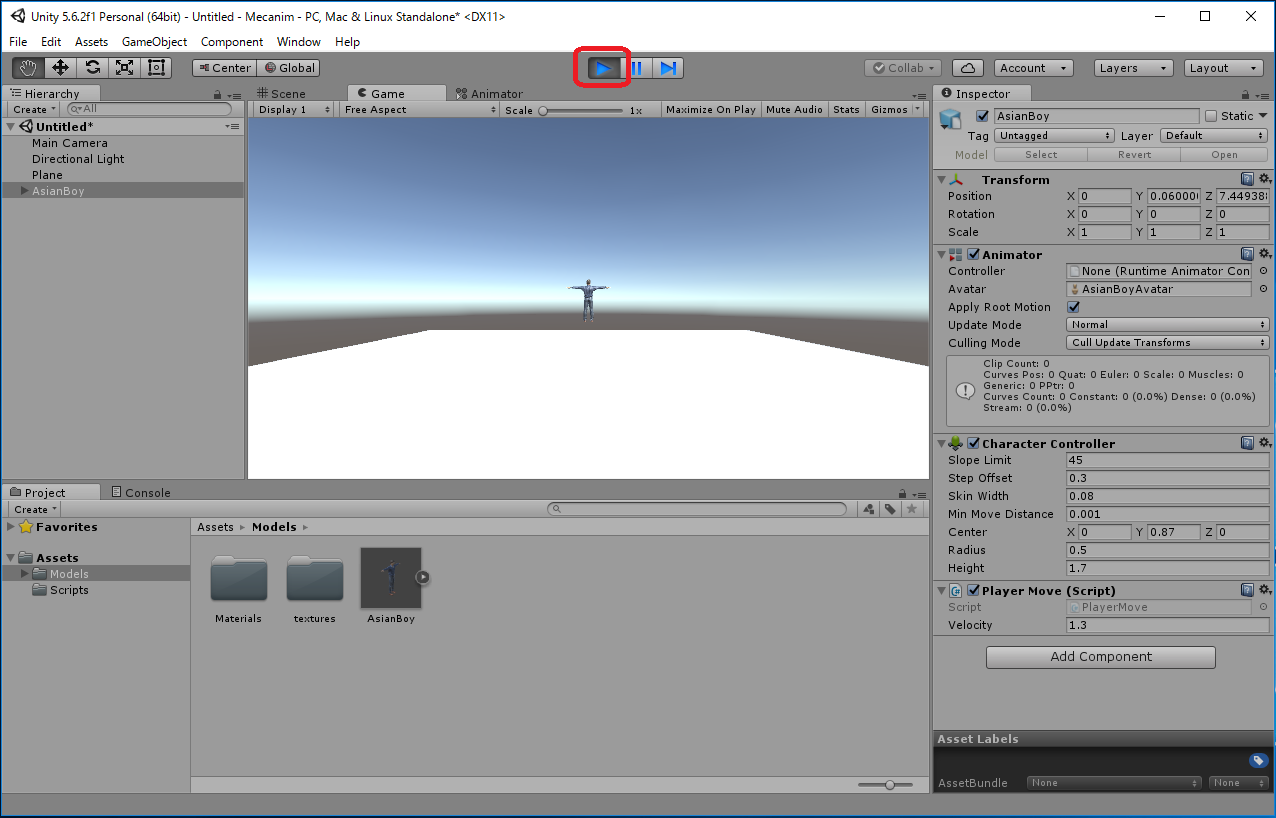
- Change the "Assets/Scripts/PlayerMove.cs" in the Project window.
When moving the Player, consider acceleration due to gravity.
It is one line to which red character part was added.
With AsianBoy moving across the edge of the Plane,
it now falls.
PlayerMove.cs |
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class PlayerMove : MonoBehaviour {
public float velocity = 1.3f;
private CharacterController charController;
void Start () {
charController = gameObject.GetComponent<CharacterController>();
}
void Update () {
float h = Input.GetAxis("Horizontal");
float v = Input.GetAxis("Vertical");
Vector3 moveDirection = new Vector3(h, 0, v);
moveDirection.y += Physics.gravity.y;
charController.Move(velocity * Time.deltaTime * moveDirection);
}
}
|
- In addition, change the "Assets/Scripts/PlayerMove.cs" in the Project window as follows.
Make it possible to move by keyboard operation only when the foot is touching the ground.
PlayerMove.cs |
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class PlayerMove : MonoBehaviour {
public float velocity = 1.3f;
private CharacterController charController;
void Start () {
charController = gameObject.GetComponent<CharacterController>();
}
void Update () {
float h = Input.GetAxis("Horizontal");
float v = Input.GetAxis("Vertical");
Vector3 moveDirection = new Vector3(0, 0, 0);;
if (charController.isGrounded) {
moveDirection = new Vector3(h, 0, v);
}
moveDirection.y += Physics.gravity.y;
charController.Move(velocity * Time.deltaTime * moveDirection);
}
}
|
- Save the Scene.
- Right click in the Project window, create the folder in which Scene will be saved.
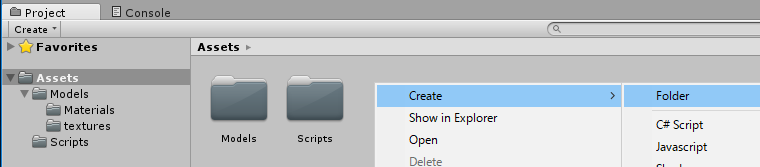
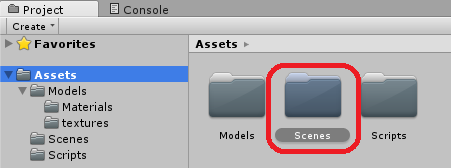
- Save the Scene as "Assets/Scenes/PlayerMove.unity".
File -> Save Scene as ... -> PlayerMove
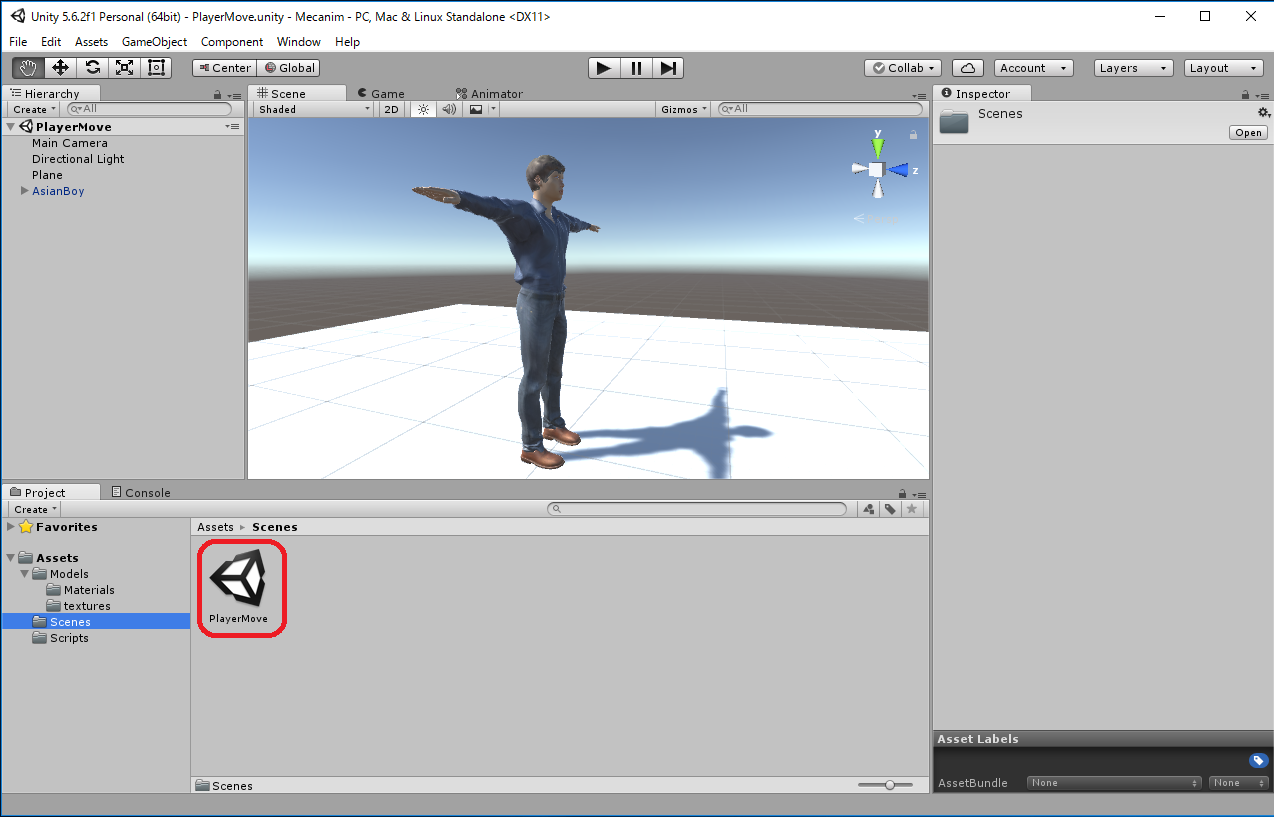
- Here is the Unity's project file Mecanim.zip which I explain here.